You can make more attractive of your display logic of model by using controllers in which, the models properties are saved to the server where as the controllers properties will not save to the server.
For instance, you can take {{name}} property rather than {{model.name}} in which Ember.js proxy properties from models. The Ember.ArrayConroller provides proxies from an array and Ember.ObjectConroller provides proxies from an object.
A Note on Coupling
The below figure shows the coupling of template, controller and model: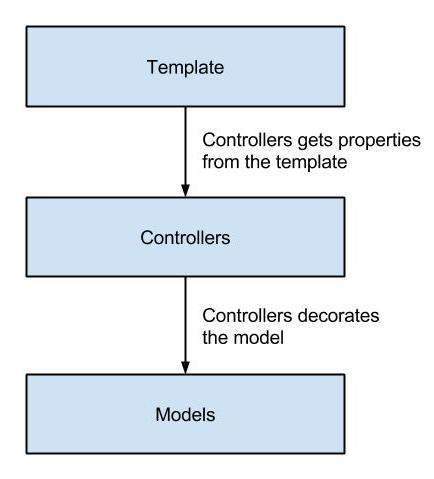
- Templates are related to the controllers and get their properties from it.
- The template deals with the controllers and controllers deals with the models.
- A model doesn't know about controllers which are decorating it and controller doesn't know about views which are representing its properties.
Representing Models
Ember.js controllers have the proxy properties, so that you can directly call the properties in your template. Templates separate properties from models and always connected to controllers, not models.For instance, you can take {{name}} property rather than {{model.name}} in which Ember.js proxy properties from models. The Ember.ArrayConroller provides proxies from an array and Ember.ObjectConroller provides proxies from an object.
Storing Application Properties
Sometimes you need save your properties of application on your server. You can store the application properties life time by storing it on a controller.Example
<!DOCTYPE html> <html> <head> <title>Emberjs Controller</title> <!-- CDN's--> <script src="https://cdnjs.cloudflare.com/ajax/libs/handlebars.js/3.0.1/handlebars.min.js"></script> <script src="https://code.jquery.com/jquery-2.1.3.min.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/ember.js/1.10.0/ember.min.js"></script> <script src="https://builds.emberjs.com/tags/v1.10.0-beta.3/ember-template-compiler.js"></script> <script src="https://builds.emberjs.com/release/ember.debug.js"></script> <script src="https://builds.emberjs.com/beta/ember-data.js"></script> </head> <body> <script type="text/x-handlebars" data-template-name="index"> <h2>Using setupController function:</h2> {{content}} </script> <script type="text/javascript"> App = Ember.Application.create(); App.IndexRoute = Ember.Route.extend({ //set up the controller and its content setupController: function(controller) { controller.set('content','Welcome to Tutorials Point'); } }); </script> </body> </html>
Output
Let's carry out the following steps to see how above code works:- Save above code in controller.htm file
- Open this HTML file in a browser.
Representing a Single Model with ObjectController
To represent the single model, you can make use of the Ember.ObjectController. Set the model property of ObjectController in the route's setupController to represent the model which you are going to use. The template looks for an ObjectController for the value of a property and the controller looks for a property with the same name in the model.Ember.ObjectController.extend({ //put your properties });In the above code, declared the ObjectController class.
Example
<!DOCTYPE html> <html> <head> <title>Emberjs Representing a Single Model with Objectcontroller</title> <!-- CDN's--> <script src="https://cdnjs.cloudflare.com/ajax/libs/handlebars.js/3.0.1/handlebars.min.js"></script> <script src="https://code.jquery.com/jquery-2.1.3.min.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/ember.js/1.10.0/ember.min.js"></script> <script src="https://builds.emberjs.com/tags/v1.10.0-beta.3/ember-template-compiler.js"></script> <script src="https://builds.emberjs.com/release/ember.debug.js"></script> <script src="https://builds.emberjs.com/beta/ember-data.js"></script> </head> <body> <script type="text/x-handlebars" data-template-name="index"> <h2>Tag:</h2> <p><b>{{tag}}<b></p> <h2>Content:</h2> <p><i>{{content}}</i>lt;/p> </script> <script type="text/javascript"> App = Ember.Application.create(); //declaring objectController with 'tag' as property and assigned value as Tutorialspoint App.IndexController = Ember.ObjectController.extend({ tag: "Tutorialspoint" }); App.IndexRoute = Ember.Route.extend({ //setting up the controller with the 'content' property setupController: function(controller) { controller.set('content','https://tutorialspoint.com'); } }); </script> </body> </html>
Output
Let's carry out the following steps to see how above code works:- Save above code in control_sing_mod.htm file
- Open this HTML file in a browser.
Representing Multiple Models with Arraycontroller
To represent the array of models, you can make use of the Ember. ArrayController . Set the model property of ArrayController in the route's setupController to represent the models which you are going to use.Ember.ArrayController.extend({ //do the logic });In the above code, declared the ArrayController class
Example
<!DOCTYPE html> <html> <head> <title>Emberjs Representing Multiple Models with Arraycontroller</title> <!-- CDN's--> <script src="https://cdnjs.cloudflare.com/ajax/libs/handlebars.js/3.0.1/handlebars.min.js"></script> <script src="https://code.jquery.com/jquery-2.1.3.min.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/ember.js/1.10.0/ember.min.js"></script> <script src="https://builds.emberjs.com/tags/v1.10.0-beta.3/ember-template-compiler.js"></script> <script src="https://builds.emberjs.com/release/ember.debug.js"></script> <script src="https://builds.emberjs.com/beta/ember-data.js"></script> </head> <body> <script type="text/x-handlebars"> <h2>Array Elements:</h2> {{#each user in controllers.users}} <li>{{user}}</li> {{/each}} </script> <script type="text/javascript"> App = Ember.Application.create(); App.ApplicationController = Ember.Controller.extend({ //needs referring the users controller needs: ['users'] }); App.UsersController = Ember.ArrayController.extend({ //users controller content: ['Mack', 'Mona', 'Manu'] }); </script> </body> </html>
Output
Let's carry out the following steps to see how above code works:- Save above code in mult_mod_arry_cntrl.htm file
- Open this HTML file in a browser.
S.N. | Array Controllers & Description |
---|---|
1 | Sorting Sorting of an array contents. |
2 | Item Controller It specifies individual items in an ArrayController while iterating over the items. |
Managing Dependencies Between Controllers
During nesting the resources you need to set the connection between the two controllers and you can also connect multiple controllers together by using an array of controller.Ember.Controller.extend({ nameofArray: ['value1', 'value2'] });In the above code, nameofArray is a custom array name given by user and value1 and value2 are the contents of the array.
Example
<!DOCTYPE html> <html> <head> <title>Emberjs Managing Dependencies Between Controllers</title> <!-- CDN's--> <script src="https://cdnjs.cloudflare.com/ajax/libs/handlebars.js/3.0.1/handlebars.min.js"></script> <script src="https://code.jquery.com/jquery-2.1.3.min.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/ember.js/1.10.0/ember.min.js"></script> <script src="https://builds.emberjs.com/tags/v1.10.0-beta.3/ember-template-compiler.js"></script> <script src="https://builds.emberjs.com/release/ember.debug.js"></script> <script src="https://builds.emberjs.com/beta/ember-data.js"></script> </head> <body> <script type="text/x-handlebars"> <!-- accessing it's parent PostController --> <b>Signed in as {{controllers.post.content}}</b> <!-- accessing it's parent UserController --> <p><b>All Users:</b></p> {{#each user in controllers.users}} <li>{{user}}</li> {{/each}} </script> <script type="text/javascript"> App = Ember.Application.create(); App.ApplicationController = Ember.Controller.extend({ needs: ['post', 'users'] }); App.UsersController = Ember.ArrayController.extend({ //Users Controller holds content as an array with 3 values content: ['Mack', 'Mona', 'Manu'] }); App.PostController = Ember.ObjectController.extend({ //Post Controller holds content property as content: 'Manu' }); </script> </body> </html>
Output
Let's carry out the following steps to see how above code works:- Save above code in contrl_mod_multi_depdency.htm file
- Open this HTML file in a browser.
No comments:
Post a Comment