Database migration is important for the following reasons −
Step 1 − Create a project named “dbProject” in xampp/wamp directory.
Step 2 − Configure the project with the appropriate database connectivity.
Step 4 − The database files migrated are stored inside the migrations directory within “app” folder.
Thus, the tables are successfully migrated.
- Database migration helps in transferring data between the specified storage types.
- Database migration refers to the context of web-based applications migrating from one platform to another.
- This process usually takes place to keep a track of data which is being outdated.
Step 1 − Create a project named “dbProject” in xampp/wamp directory.
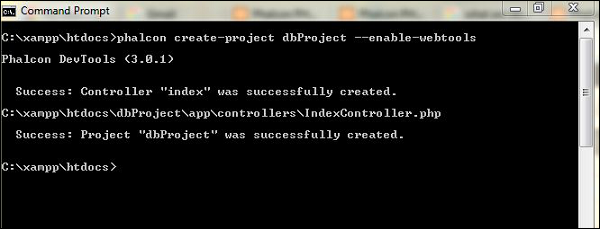
<?php /* * Modified: preppend directory path of current file, because of this file own different ENV under between Apache and command line. * NOTE: please remove this comment. */ defined('BASE_PATH') || define('BASE_PATH', getenv('BASE_PATH') ?: realpath(dirname(__FILE__) . '/../..')); defined('APP_PATH') || define('APP_PATH', BASE_PATH . '/app'); return new \Phalcon\Config(['database' => [ 'adapter' => 'Mysql', 'host' => 'localhost', 'username' => 'root', 'password' => '', 'dbname' => 'demodb', 'charset' => 'utf8', ], 'application' => [ 'appDir' => APP_PATH . '/', 'controllersDir' => APP_PATH . '/controllers/', 'modelsDir' => APP_PATH . '/models/', 'migrationsDir' => APP_PATH . '/migrations/', 'viewsDir' => APP_PATH . '/views/','pluginsDir' => APP_PATH . '/plugins/', 'libraryDir' => APP_PATH . '/library/', 'cacheDir' => BASE_PATH . '/cache/', 'baseUri' => '/dbProject/', ] ]);Step 3 − Execute the command for migration of tables included within the database “demodb”. For now, it includes one table “users”.
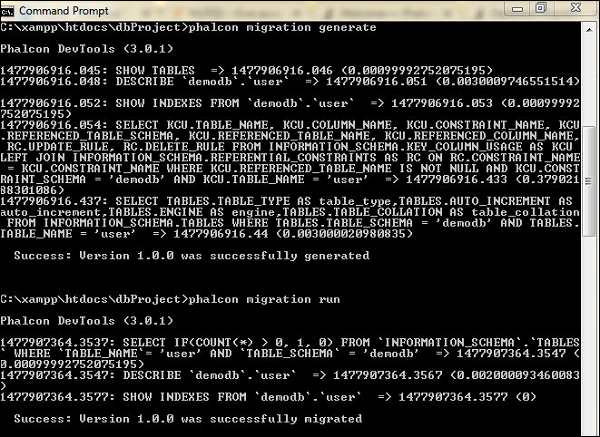

Understanding the Anatomy of Migrated Files
The migrated file has a unique class which extends Phalcon\Mvc\Model\Migration class. The Migration class in Phalcon includes the methods up() and down(). The up() method is used for performing migration while the down method rolls back the operation.Users.php
<?php use Phalcon\Db\Column; use Phalcon\Db\Index; use Phalcon\Db\Reference; use Phalcon\Mvc\Model\Migration; /** * Class UserMigration_100 */ class UserMigration_100 extends Migration { /** * Define the table structure * * @return void */ public function morph() { $this->morphTable('user', [ 'columns' => [ new Column( 'Id', [ 'type' => Column::TYPE_INTEGER, 'notNull' => true, 'autoIncrement' => true, 'size' => 11, 'first' => true ] ), new Column( 'username', [ 'type' => Column::TYPE_VARCHAR, 'notNull' => true, 'size' => 40, 'after' => 'Id' ] ), new Column( 'email', [ 'type' => Column::TYPE_VARCHAR, 'notNull' => true, 'size' => 40, 'after' => 'username' ] ), new Column( 'password', [ 'type' => Column::TYPE_VARCHAR, 'notNull' => true, 'size' => 10, 'after' => 'email' ] ) ], 'indexes' => [new Index('PRIMARY', ['Id'], 'PRIMARY') ], 'options' => [ 'TABLE_TYPE' => 'BASE TABLE', 'AUTO_INCREMENT' => '3', 'ENGINE' => 'InnoDB', 'TABLE_COLLATION' => 'latin1_swedish_ci' ], ] ); } /** * Run the migrations * * @return void */ public function up() { } /** * Reverse the migrations * * @return void */ public function down() { } }The class UserMigration_100 as shown in the example above includes associative array with four sections which are −
- Columns − Includes a set of table columns.
- Indexes − Includes a set of table indexes.
- References − Includes all the referential integrity constraints (foreign key).
- Options − Array with a set of table creation options.
No comments:
Post a Comment