State is the place where the data comes from. You should always try
to make your state as simple as possible and minimize number of stateful
components. If you have, for example, ten components that need data
from the state, you should create one container component that will keep
the state for all of them.
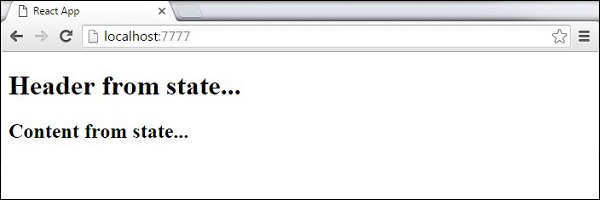
Using Props
Code sample below shows how to create stateful component using EcmaScript2016 syntax.App.jsx
import React from 'react'; class App extends React.Component { constructor(props) { super(props); this.state = { header: "Header from state...", "content": "Content from state..." } } render() { return ( <div> <h1>{this.state.header}</h1> <h2>{this.state.content}</h2> </div> ); } } export default App;
main.js
import React from 'react'; import ReactDOM from 'react-dom'; import App from './App.jsx'; ReactDOM.render(<App />, document.getElementById('app'));This will produce following result:
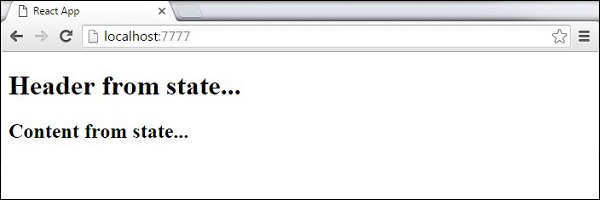
No comments:
Post a Comment