In Laravel all the exceptions are handled by app\Exceptions\Handler class. This class contains two methods — report and render.
Beside these two methods, the app\Exceptions\Handler class contains an important property called “$dontReport”. This property takes an array of exception types that will not be logged.
app/Http/routes.php
resources/views/errors/404.blade.php
http://localhost:8000/error
Step 4 − After visiting the URL, you will receive the following output −
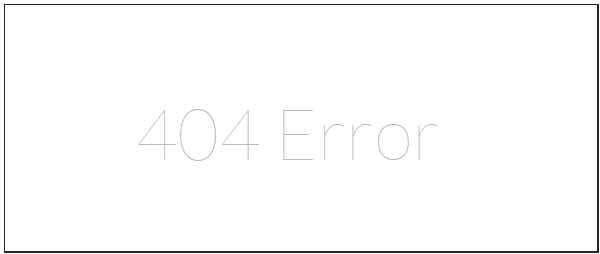
report() method
report() method is used to report or log exception. It is also used to send log exceptions to external services like Sentry, Bugsnag etc.render() method
render() method is used to render an exception into an HTTP response which will be sent back to browser.Beside these two methods, the app\Exceptions\Handler class contains an important property called “$dontReport”. This property takes an array of exception types that will not be logged.
HTTP Exceptions
Some exceptions describe HTTP error codes like 404, 500 etc. To generate such response anywhere in an application, you can use abort() method as follows.abort(404)
Custom Error pages
Laravel makes it very easy for us to use the custom error pages for each separate error codes. For example, if you want to design custom page for error code 404, you can create a view at resources/views/errors/404.blade.php. Same way, if you want to design error page for error code 500, it should be stored at resources/views/errors/500.blade.php.Example
Step 1 − Add the following lines in app/Http/routes.php.app/Http/routes.php
Route::get('/error',function(){ abort(404); });Step 2 − Create a view file called resources/views/errors/404.blade.php and copy the following code in that file.
resources/views/errors/404.blade.php
<!DOCTYPE html> <html> <head> <title>404</title> <link href = "https://fonts.googleapis.com/css?family=Lato:100" rel = "stylesheet" type = "text/css"> <style> html, body { height: 100%; } body { margin: 0; padding: 0; width: 100%; color: #B0BEC5; display: table; font-weight: 100; font-family: 'Lato'; } .container { text-align: center; display: table-cell; vertical-align: middle; } .content { text-align: center; display: inline-block; } .title { font-size: 72px; margin-bottom: 40px; } </style> </head> <body> <div class = "container"> <div class = "content"> <div class = "title">404 Error</div> </div> </div> </body> </html>Step 3 − Visit the following URL to test the event.
http://localhost:8000/error
Step 4 − After visiting the URL, you will receive the following output −
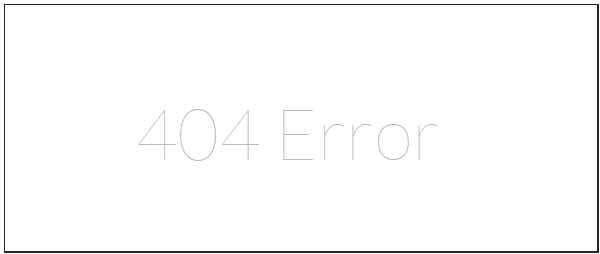
No comments:
Post a Comment