Compression is a simple, effective way to save bandwidth and speed up your site. It is only compatible with modern browsers and should be used with caution if your users use legacy browsers as well.
When sending responses from the server, if compression is used, it can greatly improve the load time. We'll be using a middleware called koa-compress to take care of compression of files as well as setting appropriate headers.
Go ahead and install the middleware using:
A response without compression:
Same response with compression:
If you look at the size tab in the bottom, you can very well see the difference between the 2. There is more the 150% improvement when we compress the files.
When sending responses from the server, if compression is used, it can greatly improve the load time. We'll be using a middleware called koa-compress to take care of compression of files as well as setting appropriate headers.
Go ahead and install the middleware using:
$ npm install --save koa-compressNow in your app.js file, add the following code:
var koa = require('koa'); var router = require('koa-router'); var app = koa(); var Pug = require('koa-pug'); var pug = new Pug({ viewPath: './views', basedir: './views', app: app //Equivalent to app.use(pug) }); app.use(compress({ filter: function (content_type) { return /text/i.test(content_type) }, threshold: 2048, flush: require('zlib').Z_SYNC_FLUSH })); var _ = router(); //Instantiate the router _.get('/', getRoot); function *getRoot(next){ this.render('index'); } app.use(_.routes()); //Use the routes defined using the router app.listen(3000);This puts our compression middleware in place. The filter option is a function that checks the response content type to decide whether to compress. The threshold option is the minimum response size in bytes to compress. This ensures we dont compress every little response.
A response without compression:
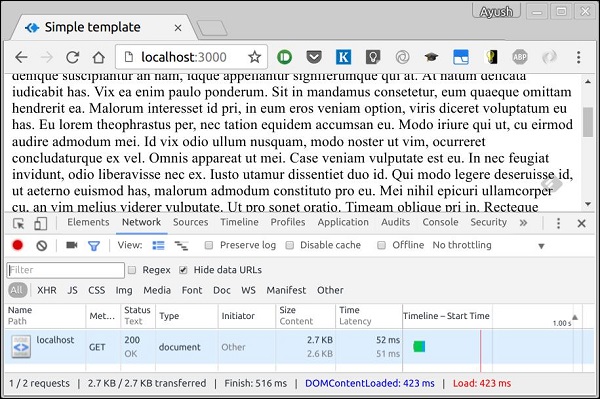

No comments:
Post a Comment