The Treeview widget displays contents of a model implementing the
gtk.TreeModel interface. PyGTK provides the following types of models −
ListStore is a list model. When associated with a gtk.TreeView widget, it produces a List box containing the items to be selected from. A gtk.ListStore object is declared with following syntax −
In order to add child rows, pass the toplevel row as parent parameter to the append() method −
Now, create a TreeView widget and use the above TreeStore object as model.
A gtk.CellRenderer is a base class for a set of objects for rendering different types of data. The derived classes are CellRendererText, CellRendererPixBuf and CellRendererToggle.
The following methods of the TreeViewColumn class are used to configure its object −
After having configured the TreeViewColumn object, it is added to the TreeView widget using the append_column() method.
The following are the important methods of the TreeView class −
Two examples of the TreeView widget are given below. The first example uses a ListStore to produce a simple ListView.
Here a ListStore object is created and string items are added to it. This ListStore object is used as model for TreeView object −
ListStore is a list model. When associated with a gtk.TreeView widget, it produces a List box containing the items to be selected from. A gtk.ListStore object is declared with following syntax −
store = gtk.ListStore(column_type)A list may have multiple columns, the predefined type constants are −
- gobject.TYPE_BOOLEAN
- gobject.TYPE_BOXED
- gobject.TYPE_CHAR
- gobject.TYPE_DOUBLE
- gobject.TYPE_ENUM
- gobject.TYPE_FLOAT
- gobject.TYPE_INT
- gobject.TYPE_LONG
- gobject.TYPE_NONE
- gobject.TYPE_OBJECT
- gobject.TYPE_STRING
- gobject.TYPE_UCHAR
- gobject.TYPE_UINT
- gobject.TYPE_ULONG
- gtk.gdk.pixbuf etc.
store = gtk.ListStore(gobject.TYPE_STRINGIn order to add items in the store, append() methods are used −
store.append (["item 1"])TreeStore is a model for multi-columned Tree widget. For example, the following statement creates a store with one column having string item.
Store = gtk.TreeStore(gobject.TYPE_STRING)In order to add items in a TreeStore, use the append() method. The append() method has two parameters, parent and row. To add toplevel item, parent is None.
row1 = store.append(None, ['row1'])You need to repeat this statement to add multiple rows.
In order to add child rows, pass the toplevel row as parent parameter to the append() method −
childrow = store.append(row1, ['child1'])You need to repeat this statement to add multiple child rows.
Now, create a TreeView widget and use the above TreeStore object as model.
treeview = gtk.TreeView(store)We now have to create TreeViewColumn to display store data. The object of gtk.TreeViewColumn manages header and the cells using gtk.CelRenderer. TreeViewColumn object is created using the following constructor −
gtk.TreeViewColumn(title, cell_renderer,…)In addition to title and renderer, it takes zero or more attribute=column pairs to specify from which tree model column the attribute's value is to be retrieved. These parameters can also be set using methods of TreeViewColumn class given below.
A gtk.CellRenderer is a base class for a set of objects for rendering different types of data. The derived classes are CellRendererText, CellRendererPixBuf and CellRendererToggle.
The following methods of the TreeViewColumn class are used to configure its object −
- TreeViewColumn.pack_start(cell, expand = True) − This method packs the CellRenderer object into the beginning column. If expand parameter is set to True, columns entire allocated space is assigned to cell.
- TreeViewColumn.add_attribute(cell, attribute, column) − This method adds an attribute mapping to the list in the tree column. The column is the column of the tree model.
- TreeViewColumn.set_attributes() − This method sets the attribute locations of the renderer using the attribute = column pairs
- TreeViewColumn.set_visible() − If True, the treeview column is visible
- TreeViewColumn.set_title() − This method sets the "title" property to the value specified.
- TreeViewColumn.set_lickable() − If set to True, the header can take keyboard focus, and be clicked.
- TreeViewColumn.set_alignment(xalign) − This method sets the "alignment" property to the value of xalign.
After having configured the TreeViewColumn object, it is added to the TreeView widget using the append_column() method.
The following are the important methods of the TreeView class −
- TreevVew.set_model() − This sets the "model" property for the treeview. If the treeview already has a model set, this method will remove it before setting the new model. If model is None, it will unset the old model.
- TreeView.set_header_clickable() − If set to True, the column title buttons can be clicked.
- TreeView.append_column() − This appends the specified TreeViewColumn to the list of columns.
- TreeView.remove_column() − This removes the specified column from the treeview.
- TreeView.insert_column() − This inserts the specified column into the treeview at the location specified by position.
cursor-changed | This is emitted when the cursor moves or is set. |
expand-collapse-cursor-row | This is emitted when the row at the cursor needs to be expanded or collapsed. |
row-activated | This is emitted when the user double clicks a treeview row |
row-collapsed | This is emitted when a row is collapsed by the user or programmatic action. |
row-expanded | This is emitted when a row is expanded via the user or programmatic action. |
Here a ListStore object is created and string items are added to it. This ListStore object is used as model for TreeView object −
store = gtk.ListStore(str) treeView = gtk.TreeView() treeView.set_model(store)Then a CellRendererText is added to a TreeViewColumn object and the same is appended to TreeView.
rendererText = gtk.CellRendererText() column = gtk.TreeViewColumn("Name", rendererText, text = 0) treeView.append_column(column)TreeView Object is placed on the toplevel window by adding it to a Fixed container.
Example 1
Observe the following code −import pygtk pygtk.require('2.0') import gtk class PyApp(gtk.Window): def __init__(self): super(PyApp, self).__init__() self.set_title("TreeView with ListStore") self.set_default_size(250, 200) self.set_position(gtk.WIN_POS_CENTER) store = gtk.ListStore(str) store.append (["PyQt"]) store.append (["Tkinter"]) store.append (["WxPython"]) store.append (["PyGTK"]) store.append (["PySide"]) treeView = gtk.TreeView() treeView.set_model(store) rendererText = gtk.CellRendererText() column = gtk.TreeViewColumn("Python GUI Libraries", rendererText, text=0) treeView.append_column(column) fixed = gtk.Fixed() lbl = gtk.Label("select a GUI toolkit") fixed.put(lbl, 25,75) fixed.put(treeView, 125,15) lbl2 = gtk.Label("Your choice is:") fixed.put(lbl2, 25,175) self.label = gtk.Label("") fixed.put(self.label, 125,175) self.add(fixed) treeView.connect("row-activated", self.on_activated) self.connect("destroy", gtk.main_quit) self.show_all() def on_activated(self, widget, row, col): model = widget.get_model() text = model[row][0] self.label.set_text(text) def main(): gtk.main() return if __name__ == "__main__": bcb = PyApp() main()The item selected by the user is displayed on a label in the window as the on_activated callback function is invoked.
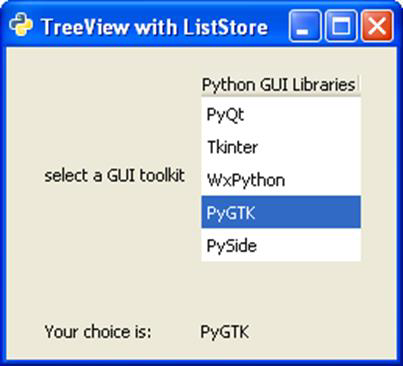
Example 2
The second example builds a hierarchical TreeView from a TreeStore. This program follows the same sequence of building the store, setting it as model for TreeView, designing a TreeViewColumn and appending it to TreeView.import gtk class PyApp(gtk.Window): def __init__(self): super(PyApp, self).__init__() self.set_title("TreeView with TreeStore") self.set_size_request(400,200) self.set_position(gtk.WIN_POS_CENTER) vbox = gtk.VBox(False, 5) # create a TreeStore with one string column to use as the model store = gtk.TreeStore(str) # add row row1 = store.append(None, ['JAVA']) #add child rows store.append(row1,['AWT']) store.append(row1,['Swing']) store.append(row1,['JSF']) # add another row row2 = store.append(None, ['Python']) store.append(row2,['PyQt']) store.append(row2,['WxPython']) store.append(row2,['PyGTK']) # create the TreeView using treestore treeview = gtk.TreeView(store) tvcolumn = gtk.TreeViewColumn('GUI Toolkits') treeview.append_column(tvcolumn) cell = gtk.CellRendererText() tvcolumn.pack_start(cell, True) tvcolumn.add_attribute(cell, 'text', 0) vbox.add(treeview) self.add(vbox) self.connect("destroy", gtk.main_quit) self.show_all() PyApp() gtk.main()The following TreeView is displayed as an output −
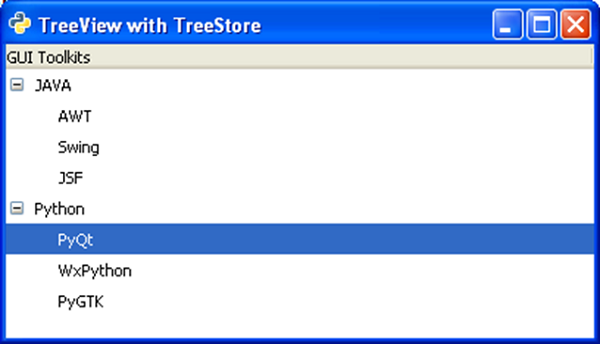
No comments:
Post a Comment