In this chapter, we are going to discuss the architectural style of
Angular 2 framework, for implementing user applications. The following
diagram shows architecture of Angular 2:
The architecture of Angular 2 contains following modules:
Some of the applications will have a component class name as AppComponent and you can find it in a file called app.component.ts. Use the export statement to export component class from module as shown below:
To register component, we use @Component annotation and can be used to break up the application into smaller parts. There will be only one component per DOM element.
For instance:
You must remember the below points while using Dependency Injection:
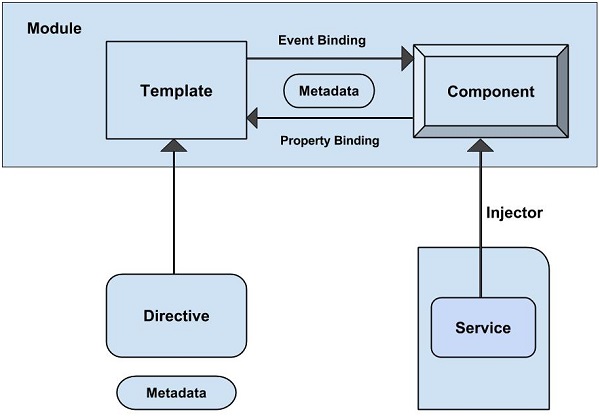
- Module
- Component
- Template
- Metadata
- Data Binding
- Service
- Directive
- Dependency Injection
Module
The module component is characterized by a block of code which can be used to perform a single task. You can export the value of something from the code such as a class. The Angular apps are called as modules and build your application using many modules. The basic building block of Angular 2 is a component class which can be exported from a module.Some of the applications will have a component class name as AppComponent and you can find it in a file called app.component.ts. Use the export statement to export component class from module as shown below:
export class AppComponent { }The export statement specifies that it is a module and its AppComponent class is defined as public and can be accessible to other modules of the application.
Component
A component is a controller class with a template which mainly deals with a view of the application and logic on the page. It is a bit of code that can be used throughout an application. Component knows how to render itself and configure dependency injection. You can associate CSS styles using component inline styles, style urls and template inline styles to a component.To register component, we use @Component annotation and can be used to break up the application into smaller parts. There will be only one component per DOM element.
Template
The component's view can be defined by using the template which tells Angular how to display the component. For instance, below simple template shows how to display the name:<div> Your name is : {{name}} </div>To display the value, you can put template expression within the interpolation braces.
Metadata
Metadata is a way of processing the class. Consider we have one component called MyComponent which will be a class until we tell Angular that it's a component. You can use metadata to the class to tell Angular that MyComponent is a component. The metadata can be attached to TypeScript by using the decorator.For instance:
@Component({ selector : 'mylist', template : '<h2>Name is Harry</h2>' directives : [MyComponentDetails] }) export class ListComponent{...}The @Component is a decorator which uses configuration object to create the component and its view. The selector creates an instance of the component where it finds <mylist> tag in parent HTML. The template tells Angular how to display the component. The directive decorator is used to represent the array of components or directives.
Data Binding
Data binding is a process of coordinating application data values by declaring bindings between sources and target HTML elements. It combines the template parts with components parts and template HTML is bound with markup to connect both sides. There are four types of data binding:- Interpolation: It displays the component value within the div tags.
- Property Binding: It passes the property from the parent to property of the child.
- Event Binding: It fires when you click on the components method name.
- Two-way Binding: This form binds property and event by using the ngModel directive in a single notation.
Service
Services are JavaScript functions that are responsible for doing a specific task only. Angular services are injected using Dependency Injection mechanism. Service includes the value, function or feature which is required by the application. Generally, service is a class which can perform something specific such as logging service, data service, message service, the configuration of application etc. There is nothing much about service in Angular and there is no ServiceBase class, but still services can be treated as fundamental to Angular application.Directive
The directive is a class that represents the metadata. There are three types of directives:- Component Directive: It creates custom controller by using view and controller and used as custom HTML element.
- Decorator Directive: It decorates the elements using additional behavior.
- Template Directive: It converts HTML into a reusable template.
Dependency Injection
Dependency Injection is a design pattern that passes an object as dependencies in different components across the application. It creates new a instance of class along with its required dependencies.You must remember the below points while using Dependency Injection:
- The Dependency Injection is stimulated into the framework and can be used everywhere.
- The injector mechanism maintains service instance and can be created using a provider.
- The provider is a way of creating a service.
- You can register the providers along with injectors.
No comments:
Post a Comment