Event aggregator should be used when your events needs to be attached
to more listeners or when you need to observe some functionality of
your app and wait for the data update. In this chapter we will show you
simple example.
Aurelia event aggregator has three methods. The publish method will fire off events and can be used by a multiple subscribers. For subscribing to an event we can use subscribe method. And finally, we can use dispose method to detach subscribers. You can see this in the following example.
Our view will just have three buttons for each of the three functionalities.
We can also detach our subscriber by clicking DISPOSE button.
Aurelia event aggregator has three methods. The publish method will fire off events and can be used by a multiple subscribers. For subscribing to an event we can use subscribe method. And finally, we can use dispose method to detach subscribers. You can see this in the following example.
Our view will just have three buttons for each of the three functionalities.
app.html
<template> <button click.delegate = "publish()">PUBLISH</button><br/> <button click.delegate = "subscribe()">SUBSCRIBE</button><br/> <button click.delegate = "dispose()">DISPOSE</button> </template>We need to import eventAggregator and inject it before we are able to use it.
app.js
import {inject} from 'aurelia-framework'; import {EventAggregator} from 'aurelia-event-aggregator'; @inject(EventAggregator) export class App { constructor(eventAggregator) { this.eventAggregator = eventAggregator; } publish(){ var payload = 'This is some data...'; this.eventAggregator.publish('myEventName', payload); } subscribe() { this.subscriber = this.eventAggregator.subscribe('myEventName', payload => { console.log(payload); }); } dispose() { this.subscriber.dispose(); console.log('Disposed!!!'); } }We need to click SUBSCRIBE button to listen for data that will be published in the future. Once the subscriber is attached, whenever new data is sent, the console will log it. If we click PUBLISH button five times, we will see that it is logged every time.
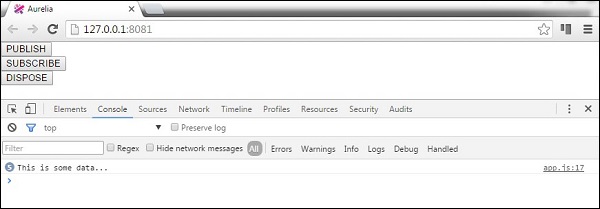
No comments:
Post a Comment