In this chapter we will show you how to use behaviors. You can think of binding behavior as a filter that can change binding data and display it in different format.
Throttle
This behavior is used to set how often should some binding update. We can use throttle to slow down rate of updating input view-model. Consider the example from our last chapter. The default rate is 200 ms. We can change that to 2 sec by adding & throttle:2000 to our input.app.js
export class App { constructor(){ this.myData = 'Enter some text!'; } }
app.html
<template> <input id = "name" type = "text" value.bind = "myData & throttle:2000" /> <h3>${myData}</h3> </template>
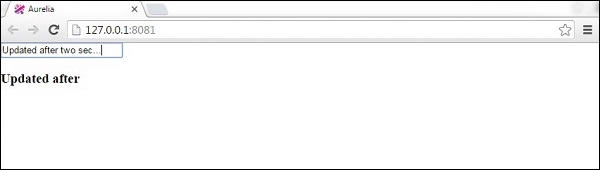
Debounce
debounce is almost the same as throttle. The difference is that debounce will update binding after user stopped typing. Example below will update binding if user stops typing for two seconds.app.js
export class App { constructor(){ this.myData = 'Enter some text!'; } }
app.html
<template> <input id = "name" type = "text" value.bind = "myData & debounce:2000" /> <h3>${myData}</h3> </template>
oneTime
oneTime is the most efficient behavior performance wise. You should always use it when you know that data should be bound only once.app.js
export class App { constructor(){ this.myData = 'Enter some text!'; } }
app.html
<template> <input id = "name" type = "text" value.bind = "myData & oneTime" /> <h3>${myData}</h3> </template>The last example above will bind text to the view, but if we change the default text, nothing will happen since it is bound only once.
No comments:
Post a Comment