Fortran - Overview
Fortran, as derived from Formula Translating System, is a general-purpose, imperative programming language. It is used for numeric and scientific computing.Fortran was originally developed by IBM in the 1950s for scientific and engineering applications. Fortran ruled this programming area for a long time and became very popular for high performance computing, because.
It supports:
- Numerical analysis and scientific computation
- Structured programming
- Array programming
- Modular programming
- Generic programming
- High performance computing on supercomputers
- Object oriented programming
- Concurrent programming
- Reasonable degree of portability between computer systems
Facts about Fortran
- Fortran was created by a team, led by John Backus at IBM in 1957.
- Initially the name used to be written in all capital, but current standards and implementations only require the first letter to be capital.
- Fortran stands for FORmula TRANslator.
- Originally developed for scientific calculations, it had very limited support for character strings and other structures needed for general purpose programming.
- Later extensions and developments made it into a high level programming language with good degree of portability.
- Original versions, Fortran I, II and III are considered obsolete now.
- Oldest version still in use is Fortran IV, and Fortran 66.
- Most commonly used versions today are : Fortran 77, Fortran 90, and Fortran 95.
- Fortran 77 added strings as a distinct type.
- Fortran 90 added various sorts of threading, and direct array processing.
Fortran - Environment Setup
Setting up Fortran in Windows
G95 is the GNU Fortran multi-architechtural compiler, used for setting up Fortran in Windows. The windows version emulates a unix environment using MingW under windows. The installer takes care of this and automatically adds g95 to the windows PATH variable.You can get the stable version of G95 from here :

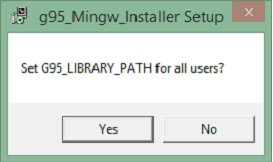
How to use G95
During installation, g95 is automatically added to your PATH variable if you select the option “RECOMMENDED”. This means that you can simply open a new Command Prompt window and type “g95” to bring up the compiler. Find some basic commands below to get you started.Command | Description |
---|---|
g95 –c hello.f90 | Compiles hello.f90 to an object file named hello.o |
g95 hello.f90 | Compiles hello.f90 and links it to produce an executable a.out |
g95 -c h1.f90 h2.f90 h3.f90 | Compiles multiple source files. If all goes well, object files h1.o, h2.o and h3.o are created |
g95 -o hello h1.f90 h2.f90 h3.f90 | Compiles multiple source files and links them together to an executable file named 'hello' |
-c Compile only, do not run the linker. -o Specify the name of the output file, either an object file or the executable.Multiple source and object files can be specified at once. Fortran files are indicated by names ending in ".f", ".F", ".for", ".FOR", ".f90", ".F90", ".f95", ".F95", ".f03" and ".F03". Multiple source files can be specified. Object files can be specified as well and will be linked to form an executable file.
Fortran - Basic Syntax
A Fortran program is made of a collection of program units like a main program, modules, and external subprograms or procedures.Each program contains one main program and may or may not contain other program units. The syntax of the main program is as follows:
program program_name implicit none ! type declaration statements ! executable statements end program program_name
A Simple Program in Fortran
Let’s write a program that adds two numbers and prints the result:program addNumbers ! This simple program adds two numbers implicit none ! Type declarations real :: a, b, result ! Executable statements a = 12.0 b = 15.0 result = a + b print *, 'The total is ', result end program addNumbersWhen you compile and execute the above program, it produces the following result:
The total is 27.0000000Please note that:
- All Fortran programs start with the keyword program and end with the keyword end program, followed by the name of the program.
- The implicit none statement allows the compiler to check that all your variable types are declared properly. You must always use implicit none at the start of every program.
- Comments in Fortran are started with the exclamation mark (!), as all characters after this (except in a character string) are ignored by the compiler.
- The print * command displays data on the screen.
- Indentation of code lines is a good practice for keeping a program readable.
- Fortran allows both uppercase and lowercase letters. Fortran is case-insensitive, except for string literals.
Basics
The basic character set of Fortran contains:- the letters A ... Z and a ... z
- the digits 0 ... 9
- the underscore (_) character
- the special characters = : + blank - * / ( ) [ ] , . $ ' ! " % & ; < > ?
Program statements are made of tokens.
Identifier
An identifier is a name used to identify a variable, procedure, or any other user-defined item. A name in Fortran must follow the following rules:- It cannot be longer than 31 characters.
- It must be composed of alphanumeric characters (all the letters of the alphabet, and the digits 0 to 9) and underscores (_).
- First character of a name must be a letter.
- Names are case-insensitive
Keywords
Keywords are special words, reserved for the language. These reserved words cannot be used as identifiers or names.The following table, lists the Fortran keywords:
The non-I/O keywords | ||||
---|---|---|---|---|
allocatable | allocate | assign | assignment | block data |
call | case | character | common | complex |
contains | continue | cycle | data | deallocate |
default | do | double precision | else | else if |
elsewhere | end block data | end do | end function | end if |
end interface | end module | end program | end select | end subroutine |
end type | end where | entry | equivalence | exit |
external | function | go to | if | implicit |
in | inout | integer | intent | interface |
intrinsic | kind | len | logical | module |
namelist | nullify | only | operator | optional |
out | parameter | pause | pointer | private |
program | public | real | recursive | result |
return | save | select case | stop | subroutine |
target | then | type | type() | use |
Where | While | |||
The I/O related keywords | ||||
backspace | close | endfile | format | inquire |
open | read | rewind | Write |
Fortran - Data Types
Fortran provides five intrinsic data types, however, you can derive your own data types as well. The five intrinsic types are:- Integer type
- Real type
- Complex type
- Logical type
- Character type
Integer Type
The integer types can hold only integer values. The following example extracts the largest value that can be held in a usual four byte integer:program testingInt implicit none integer :: largeval print *, huge(largeval) end program testingIntWhen you compile and execute the above program it produces the following result:
2147483647
Note that the huge() function gives the largest number that
can be held by the specific integer data type. You can also specify the
number of bytes using the kind specifier. The following example demonstrates this:program testingInt implicit none !two byte integer integer(kind=2) :: shortval !four byte integer integer(kind=4) :: longval !eight byte integer integer(kind=8) :: verylongval !sixteen byte integer integer(kind=16) :: veryverylongval !default integer integer :: defval print *, huge(shortval) print *, huge(longval) print *, huge(verylongval) print *, huge(veryverylongval) print *, huge(defval) end program testingIntWhen you compile and execute the above program, it produces the following result:
32767 2147483647 9223372036854775807 170141183460469231731687303715884105727
No comments:
Post a Comment