The following example show how to write a simple web based application which makes use of redirect
to transfer a http request to another another page. To start with it,
let us have working Eclipse IDE in place and follow the following steps
to develop a Dynamic Form based Web Application using Spring Web
Framework:
WebController.java
index.jsp
Now start your Tomcat server and make sure you are able to access other web pages from webapps folder using a standard browser. Now try a URL http://localhost:8080/HelloWeb/index and you should see the following result if everything is fine with your Spring Web Application:
Now click on "Redirect Page" button to submit the form and to get
final redirected page. You should see the following result if everything
is fine with your Spring Web Application:
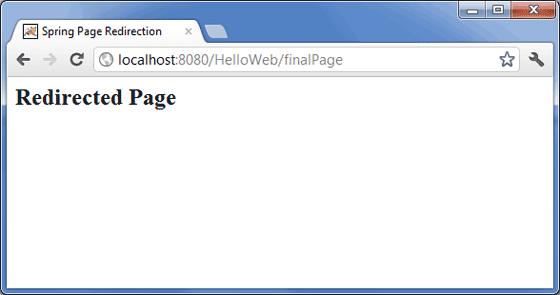
Step | Description |
---|---|
1 | Create a project with a name HelloWeb under a package com.tutorialspoint as explained in the Spring MVC - Hello World Example chapter. |
2 | Create a Java class WebController under the com.tutorialspoint package. |
3 | Create a view files index.jsp, final.jsp under jsp sub-folder. |
4 | The final step is to create the content of all the source and configuration files and export the application as explained below. |
package com.tutorialspoint; import org.springframework.stereotype.Controller; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestMethod; @Controller public class WebController { @RequestMapping(value = "/index", method = RequestMethod.GET) public String index() { return "index"; } @RequestMapping(value = "/redirect", method = RequestMethod.GET) public String redirect() { return "redirect:finalPage"; } @RequestMapping(value = "/finalPage", method = RequestMethod.GET) public String finalPage() { return "final"; } }Following is the content of Spring view file index.jsp. This will be a landing page, this page will send a request to access redirect service method which will redirect this request to another service method and finally a final.jsp page will be displayed.
index.jsp
<%@taglib uri="http://www.springframework.org/tags/form" prefix="form"%> <html> <head> <title>Spring Page Redirection</title> </head> <body> <h2>Spring Page Redirection</h2> <p>Click below button to redirect the result to new page</p> <form:form method="GET" action="/HelloWeb/redirect"> <table> <tr> <td> <input type="submit" value="Redirect Page"/> </td> </tr> </table> </form:form> </body> </html>final.jsp
<%@taglib uri="http://www.springframework.org/tags/form" prefix="form"%> <html> <head> <title>Spring Page Redirection</title> </head> <body> <h2>Redirected Page</h2> </body> </html>Once you are done with creating source and configuration files, export your application. Right click on your application and use Export > WAR File option and save your HelloWeb.war file in Tomcat's webapps folder.
Now start your Tomcat server and make sure you are able to access other web pages from webapps folder using a standard browser. Now try a URL http://localhost:8080/HelloWeb/index and you should see the following result if everything is fine with your Spring Web Application:
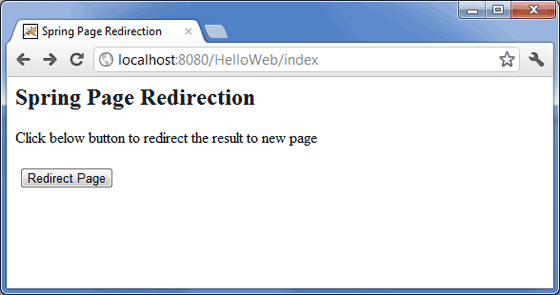
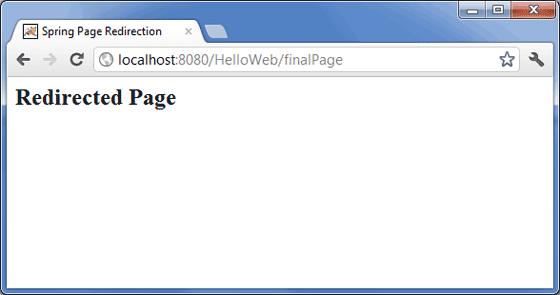
No comments:
Post a Comment