Erlang is a functional programming language which also has a runtime
environment. It was built in such a way that it had integrated support
for concurrency, distribution and fault tolerance. Erlang was originally
developed to be used in several large telecommunication systems from
Ericsson.
Labels
.
Search Your Article
Total Pageviews
Tuesday, January 31, 2017
Erlang - Environment
Try it Option Online
You really do not need to set up your own environment to start learning Erlang programming language. Reason is very simple, we have already set up Erlang Programming environment online, so that you can compile and execute all the available examples online at the same time when you are doing your theory work.
Erlang - Basic Syntax
In order to understand the basic syntax of Erlang, let’s first look at a simple Hello World program.
Example
% hello world program -module(helloworld). -export([start/0]). start() ->
Erlang - Shell
The Erlang shell is used for testing of expressions. Hence, testing
can be carried out in the shell very easily before it actually gets
tested in the application itself.
The following example showcases how the addition expression can be used in the shell. What needs to be noted here is that the expression needs to end with the dot (.) delimiter.
The following example showcases how the addition expression can be used in the shell. What needs to be noted here is that the expression needs to end with the dot (.) delimiter.
Erlang - Data Types
In any programming language, you need to use several variables to
store various types of information. Variables are nothing but reserved
memory locations to store values. This means that when you create a
variable you reserve some space in the memory to store the value
associated with that variable.
Erlang - Variables
In Erlang, all the variables are bound with the ‘=’ statement. All
variables need to start with the upper case character. In other
programming languages, the ‘=’ sign is used for the assignment, but not
in the case of Erlang. As stated, variables are defined with the use of
the ‘=’ statement.
Erlang - Operators
An operator is a symbol that tells the compiler to perform specific mathematical or logical manipulations.
Erlang has the following type of operators −
Erlang has the following type of operators −
- Arithmetic operators
- Relational operators
- Logical operators
- Bitwise operators
Erlang - Loops
Erlang is a functional programming language and what needs to be
remembered about all functional programming languages is that they don’t
offer any constructs for loops. Instead, functional programming depends
on a concept called recursion.
Erlang - Decision Making
Decision making structures requires that the programmer should
specify one or more conditions to be evaluated or tested by the program,
along with a statement or statements to be executed if the condition is
determined to be true, and optionally, other statements to be executed if the condition is determined to be false.
Erlang - Functions
Erlang is known as a functional programming language, hence you would
expect to see a lot of emphasis on how functions work in Erlang. This
chapter covers what all can be done with the functions in Erlang.
Erlang - Modules
Modules are a bunch of functions regrouped in a single file, under a
single name. Additionally, all functions in Erlang must be defined in
modules.
Most of the basic functionality like arithmetic, logic and Boolean operators are already available because the default modules are loaded when a program is run.
Most of the basic functionality like arithmetic, logic and Boolean operators are already available because the default modules are loaded when a program is run.
Erlang - Recursion
Recursion is an important part of Erlang. First let’s see how we can
implement simple recursion by implementing the factorial program.
Erlang - Numbers
In Erlang there are 2 types of numeric literals which are integers
and floats. Following are some examples which show how integers and
floats can be used in Erlang.
Integer − An example of how the number data type can be used as an integer is shown in the following program. This program shows the addition of 2 Integers.
Integer − An example of how the number data type can be used as an integer is shown in the following program. This program shows the addition of 2 Integers.
Erlang - Strings
A String literal is constructed in Erlang by enclosing the string
text in quotations. Strings in Erlang need to be constructed using the
double quotation marks such as “Hello World”.
Following is an example of the usage of strings in Erlang −
Following is an example of the usage of strings in Erlang −
Erlang - Lists
The List is a structure used to store a collection of data items. In
Erlang, Lists are created by enclosing the values in square brackets.
Following is a simple example of creating a list of numbers in Erlang.
Following is a simple example of creating a list of numbers in Erlang.
Erlang - File I/O
Erlang provides a number of methods when working with I/O. It has
easier classes to provide the following functionalities for files −
Erlang - Atoms
An atom is a literal, a constant with name. An atom is to be enclosed
in single quotes (') if it does not begin with a lower-case letter or
if it contains other characters than alphanumeric characters, underscore
(_), or @.
The following program is an example of how atoms can be used in Erlang. This program declares 3 atoms, atom1, atom_1 and ‘atom 1’ respectively. So you can see the different ways an atom can be declared.
The following program is an example of how atoms can be used in Erlang. This program declares 3 atoms, atom1, atom_1 and ‘atom 1’ respectively. So you can see the different ways an atom can be declared.
Erlang - Maps
A map is a compound data type with a variable number of key-value
associations. Each key-value association in the map is called an
association pair. The key and value parts of the pair are called
elements. The number of association pairs is said to be the size of the
map.
Erlang - Tuples
A tuple is a compound data type with a fixed number of terms. Each
term in the Tuple is called an element. The number of elements is said
to be the size of the Tuple.
An example of how the Tuple data type can be used is shown in the following program.
An example of how the Tuple data type can be used is shown in the following program.
Erlang - Records
Erlang has the extra facility to create records. These records
consist of fields. For example, you can define a personal record which
has 2 fields, one is the id and the other is the name field. In Erlang,
you can then create various instances of this record to define multiple
people with various names and id’s.
Let’s explore how we can work with records.
Let’s explore how we can work with records.
Erlang - Exceptions
Exception handling is required in any programming language to handle
the runtime errors so that normal flow of the application can be
maintained. Exception normally disrupts the normal flow of the
application, which is the reason why we need to use Exception handling
in our application.
Erlang - Macros
Macros are generally used for inline code replacements. In Erlang, macros are defined via the following statements
- -define(Constant, Replacement).
- -define(Func(Var1, Var2,.., Var), Replacement).
Erlang - Header Files
Header files are like include files in any other programming
language. It is useful for splitting modules into different files and
then accessing these header files into separate programs. To see header
files in action, let’s look at one of our earlier examples of records.
Erlang - Preprocessors
Before an Erlang module is compiled, it is automatically processed by
the Erlang Preprocessor. The preprocessor expands any macros that might
be in the source file and inserts any necessary include files.
Ordinarily, you won’t need to look at the output of the preprocessor, but in exceptional circumstances (for example, when debugging a faulty macro), you might want to save the output of the preprocessor.
Ordinarily, you won’t need to look at the output of the preprocessor, but in exceptional circumstances (for example, when debugging a faulty macro), you might want to save the output of the preprocessor.
Erlang - Pattern Matching
Patterns look the same as terms – they can be simple literals like
atoms and numbers, compound like tuples and lists, or a mixture of both.
They can also contain variables, which are alphanumeric strings that
begin with a capital letter or underscore. A special "anonymous
variable", _ (the underscore) is used when you don't care about the
value to be matched, and won't be using it.
Erlang - Guards
Guards are constructs that we can use to increase the power of
pattern matching. Using guards, we can perform simple tests and
comparisons on the variables in a pattern.
The general syntax of the guard statement is as follows −
The general syntax of the guard statement is as follows −
function(parameter) when condition ->Where,
Erlang - BIFS
BIFs are functions that are built into Erlang. They usually do tasks
that are impossible to program in Erlang. For example, it’s impossible
to turn a list into a tuple or to find the current time and date. To
perform such an operation, we call a BIF.
Erlang - Binaries
Use a data structure called a binary to store large quantities of raw
data. Binaries store data in a much more space efficient manner than in
lists or tuples, and the runtime system is optimized for the efficient
input and output of binaries.
Erlang - Funs
Funs are used to define anonymous functions in Erlang. The general syntax of an anonymous function is given below
Syntax
F = fun (Arg1, Arg2, ... ArgN) -> ... EndWhere
Erlang - Processes
The granularity of concurrency in Erlang is a process. A process is
an activity/task that runs concurrently with and is independent from the
other processes. These processes in Erlang are different than the
processes and threads most people are familiar with. Erlang processes
are lightweight, operate in (memory) isolation from other processes, and
are scheduled by Erlang’s Virtual Machine (VM).
Erlang - Email
To send an email using Erlang, you need to use a package available from github for the same. The github link is – https://github.com/Vagabond/gen_smtp
This link contains an smtp utility which can be used for sending email from an Erlang application. Follow the steps to have the ability to send an email from Erlang
This link contains an smtp utility which can be used for sending email from an Erlang application. Follow the steps to have the ability to send an email from Erlang
Erlang - Databases
Erlang has the ability to connect to the traditional databases such as SQL Server and Oracle. Erlang has an inbuilt odbc library that can be used to work with databases.
Erlang - Ports
In Erlang, ports are used for communication between different
programs. A socket is a communication endpoint that allows machines to
communicate over the Internet by using the Internet Protocol (IP).
Erlang - Distributed Programming
Distributed Programs are those programs that are designed to run on
networks of computers and that can coordinate their activities only by
message passing.
There are a number of reasons why we might want to write distributed applications. Here are some of them.
There are a number of reasons why we might want to write distributed applications. Here are some of them.
Erlang - OTP
OTP stands for Open Telecom Platform. It’s an application operating
system and a set of libraries and procedures used for building
large-scale, fault-tolerant, distributed applications. If you want to
program your own applications using OTP, then the central concept that
you will find very useful is the OTP behavior. A behavior encapsulates
common behavioral patterns — think of it as an application framework
that is parameterized by a callback module.
Erlang - Concurrency
Concurrent programming in Erlang needs to have the following basic principles or processes.
The list includes the following principles −
The list includes the following principles −
piD = spawn(Fun)
Creates a new concurrent process that evaluates Fun. The new process runs in parallel with the caller. An example is as follows −Erlang - Performance
When talking about performance the following points need to be noted about Erlang.
- Funs are very fast − Funs was given its own data type in R6B and was further optimized in R7B.
- Using the ++ operator − This operator needs to be used in the proper way. The following example is the wrong way to do a ++ operation.
Erlang - Drivers
Sometimes we want to run a foreign-language program inside the Erlang
Runtime System. In this case, the program is written as a shared
library that is dynamically linked into the Erlang runtime system. The
linked-in driver appears to the programmer as a port program and obeys
exactly the same protocol as for a port program.
Erlang - Web Programming
In Erlang, the inets library is available to build web servers
in Erlang. Let’s look at some of the functions available in Erlang for
web programming. One can implement the HTTP server, also referred to as
httpd to handle HTTP requests.
The server implements numerous features, such as −
The server implements numerous features, such as −
Erlang - Quick Guide
Erlang - Overview
Erlang is a functional programming language which also has a runtime environment. It was built in such a way that it had integrated support for concurrency, distribution and fault tolerance. Erlang was originally developed to be used in several large telecommunication systems from Ericsson.Erlang - Useful Resources
The following resources contain additional information on Erlang. Please use them to get more in-depth knowledge on this.
Discuss Erlang
Erlang is a general purpose or you might say a functional programming
language and runtime environment. It was built in such a way that it had
inherent support for concurrency, distribution and fault tolerance.
Erlang was originally developed to be used in several large
telecommunication systems. But it has now slowly made its foray into
diverse sectors like ecommerce, computer telephony and banking sectors
as well.
Elixir - Overview
Elixir is a dynamic, functional language designed for building
scalable and maintainable applications.
Elixir leverages the Erlang VM, known for running low-latency,
distributed and fault-tolerant systems, while also being successfully
used in web development and the embedded software domain.
Elixir - Environment
In order to run Elixir, you need to set it up locally on your system.
To install Elixir, you'll first need erlang. On some platforms, elixir
packages come with erlang in them.
Elixir - Basic Syntax
We'll start with the customary 'Hello World' program. Start the elixir interactive shell using
iexAfter the shell starts, use the IO.puts function to "put" the string on the console output. Enter the following in your elixir shell:
IO.puts "Hello world"
Elixir - Data Types
For using any language, you need to understand the basic data types
the language supports. In this chapter, we will discuss 7 basic data
types supported by the elixir language: integers, floats, booleans,
atoms, strings, lists and tuples.
Elixir - Variables
A variable provides us with named storage that our programs can
manipulate. Each variable in Elixir has a specific type, which
determines the size and layout of the variable's memory; the range of
values that can be stored within that memory; and the set of operations
that can be applied to the variable.
Elixir supports the following basic types of variables:
Elixir supports the following basic types of variables:
Elixir - Operators
An operator is a symbol that tells the compiler to perform specific
mathematical or logical manipulations. There are a LOT of operators
provided by elixir. They are divided in the following categories:
- Arithmetic operators
- Comparison operators
- Boolean operators
- Misc operators
Elixir - Pattern Matching
Pattern matching is a technique which Elixir inherits form Erlang.
Pattern matching is a very powerful technique that allows us to extract
simpler substructures from complicated data structures like lists,
tuples, maps, etc.
Elixir - Decision Making
Decision making structures require that the programmer specifies one
or more conditions to be evaluated or tested by the program, along with a
statement or statements to be executed if the condition is determined
to be true, and optionally, other statements to be executed if the condition is determined to be false.
Following is the general from of a typical decision making structure found in most of the programming language:
Following is the general from of a typical decision making structure found in most of the programming language:
Elixir - Strings
Strings in Elixir are inserted between double quotes, and they are encoded in UTF-8. Unlike C and C++ where default strings are ASCII encoded and only 256 different characters are possible, UTF-8 consists of 66536 code points. This means that UTF-8 encoding consists of those many different possible characters. Since the strings use utf-8, we can also use symbols like: ö, ł, etc.
Elixir - Char lists
A char list is nothing more than a list of characters. For example:
IO.puts('Hello') IO.puts(is_list('Hello'))When running above program, it produces following result:
Elixir - Lists and Tuples
(Linked) Lists
A linked list is a heterogeneous list of elements that are stored at different locations in memory and are kept track of by using references. Linked lists are very useful data structures especially used a lot in functional programming.Elixir - Keyword lists
So far we haven’t discussed any associative data structures, i.e. data structures that are able to associate a certain value (or multiple values) to a key. Different languages call these different names like dictionaries, hashes, associative arrays, etc.
Elixir - Maps
Keyword lists are a convenient way to address content stored in lists by key, but underneath, Elixir is still walking through the list. That might be OK if you have other plans for that list requiring walking through all of it, but it can be unnecessary overhead if you’re planning to use keys as your only approach to the data.
This is where maps come to your rescue. Whenever you need a key-value store, maps are the “go to” data structure in Elixir.
This is where maps come to your rescue. Whenever you need a key-value store, maps are the “go to” data structure in Elixir.
Monday, January 30, 2017
Elixir - Modules
In Elixir we group several functions into modules. We’ve already used
many different modules in the previous chapters such as the String
module, Bitwise module, Tuple module, etc.
In order to create our own modules in Elixir, we use the defmodule macro. We use the def macro to define functions in that module:
In order to create our own modules in Elixir, we use the defmodule macro. We use the def macro to define functions in that module:
Elixir - Aliases
In order to facilitate software reuse, Elixir provides three directives (alias, require and import) plus a macro called use summarized below:
# Alias the module so it can be called as Bar instead of Foo.Bar alias Foo.Bar, as: Bar # Ensure the module is compiled and available (usually for macros) require Foo
Elixir - Functions
A function is a set of statements organized together to perform a
specific task. Functions in programming work mostly like function in
math. You give functions some input, they generate output based on the
input provided. There are 2 types of functions in elixir:
Elixir - Recursion
Recursion is a method where the solution to a problem depends on
solutions to smaller instances of the same problem. Most computer
programming languages support recursion by allowing a function to call
itself within the program text.
Elixir - Loops
Due to immutability, loops in Elixir (as in any functional
programming language) are written differently from imperative languages.
For example, in an imperative language like C, you would write:
for(i = 0; i < 10; i++) { printf("%d", array[i]);
Elixir - Enumerables
An enumerable is an object that may be enumerated. "Enumerated" means
to count off the members of a set/collection/category one by one
(usually in order, usually by name).
Elixir provides the concept of enumerables and the Enum module to work with them. The functions in the Enum module are limited to, as the name says, enumerating values in data structures.
Elixir provides the concept of enumerables and the Enum module to work with them. The functions in the Enum module are limited to, as the name says, enumerating values in data structures.
Elixir - Streams
All the functions in the Enum module are eager. Many functions expect
an enumerable and return a list back. This means that when performing
multiple operations with Enum, each operation is going to generate an
intermediate list until we reach the result. We saw an example for the
same in the previous chapter.
Elixir - Structs
Structs are extensions built on top of maps that provide compile-time checks and default values.
Defining structs
To define a struct, the defstruct construct is used:defmodule User do defstruct name: "John", age: 27 end
Elixir - Protocols
Protocols are a mechanism to achieve polymorphism in Elixir.
Dispatching on a protocol is available to any data type as long as it
implements the protocol. Lets look at an example of using protocols. We
used a function called to_string in the previous chapters to
convert from other types to the string type.
Elixir - File IO
File IO is an integral part of any programming language as it allows
the language to interact with the files on the file system. In this
chapter we'll have a look at 2 modules: Path and File.
Elixir - Processes
In Elixir, all code runs inside processes. Processes are isolated
from each other, run concurrent to one another and communicate via
message passing. Elixir’s processes should not be confused with
operating system processes. Processes in Elixir are extremely
lightweight in terms of memory and CPU (unlike threads in many other
programming languages). Because of this, it is not uncommon to have tens
or even hundreds of thousands of processes running simultaneously.
Elixir - Sigils
In this chapter, we are going to explore sigils, which are one of the
mechanisms provided by the language for working with textual
representations. Sigils start with the tilde (~) character which is
followed by a letter (which identifies the sigil) and then a delimiter;
optionally, modifiers can be added after the final delimiter.
Elixir - Comprehensions
List comprehensions are syntactic sugar for looping through
enumerables in Elixir. In this chapter we'll use comprehensions for
iteration and generation.
Elixir - Typespecs
Elixir is a dynamically typed language, so all types in Elixir are
inferred by the runtime. Nonetheless, Elixir comes with typespecs, which
are a notation used for declaring custom data types and declaring typed function signatures (specifications).
Elixir - Behaviours
Behaviours in Elixir (and Erlang) are a way to separate and abstract
the generic part of a component (which becomes the behaviour module)
from the specific part (which becomes the callback module). Behaviours
provide a way to:
Elixir - Error Handling
Elixir has three error mechanisms: errors, throws and exits. Let us explore each of them.
Error
Errors (or exceptions) are used when exceptional things happen in the code. A sample error can be retrieved by trying to add a number into an string:IO.puts(1 + "Hello")
Elixir - Macros
Macros are one of the most advanced and powerful features of Elixir.
As with all advanced features of any language, macros shoild be used
sparingly. They make it possible to perform powerful code
transformations in compile time. Macros are a fairly complex subject,
and it would take a small tutorial to explain it in depth. Here we'll
just scratch the surface and get familiar with what macros are and how
to use them.
Elixir - Libraries
Elixir provides excellent interoperability with Erlang libraries. Let us have a look at some of these libraries.
The binary module
The built-in Elixir String module handles binaries that are UTF-8 encoded. The binary module is useful when you are dealing with binary data that is not necessarily UTF-8 encoded. Let us look at an example:# UTF-8 IO.puts(String.to_char_list("Ø"))
Data Structures & Algorithms - Overview
Data Structure is a systematic way to organize data in order to use
it efficiently. Following terms are the foundation terms of a data
structure.
Data Structures - Environment Setup
Try it Option Online
You really do not need to set up your own environment to start learning C programming language. Reason is very simple, we already have set up C Programming environment online, so that you can compile and execute all the available examples online at the same time when you are doing your theory work.
Data Structures - Algorithms Basics
Algorithm is a step-by-step procedure, which defines a set of
instructions to be executed in a certain order to get the desired
output. Algorithms are generally created independent of underlying
languages, i.e. an algorithm can be implemented in more than one
programming language.
Data Structures - Asymptotic Analysis
Asymptotic analysis of an algorithm refers to defining the
mathematical boundation/framing of its run-time performance. Using
asymptotic analysis, we can very well conclude the best case, average
case, and worst case scenario of an algorithm.
Data Structures - Greedy Algorithms
An algorithm is designed to achieve optimum solution for a given
problem. In greedy algorithm approach, decisions are made from the given
solution domain. As being greedy, the closest solution that seems to
provide an optimum solution is chosen.
Data Structures - Divide and Conquer
In divide and conquer approach, the problem in hand, is divided into
smaller sub-problems and then each problem is solved independently. When
we keep on dividing the subproblems into even smaller sub-problems, we
may eventually reach a stage where no more division is possible.
Data Structures - Dynamic Programming
Dynamic programming approach is similar to divide and conquer in
breaking down the problem into smaller and yet smaller possible
sub-problems. But unlike, divide and conquer, these sub-problems are not
solved independently. Rather, results of these smaller sub-problems are
remembered and used for similar or overlapping sub-problems.
Data Structures & Algorithm Basic Concepts
This chapter explains the basic terms related to data structure.
Data Definition
Data Definition defines a particular data with the following characteristics.- Atomic − Definition should define a single concept.
- Traceable − Definition should be able to be mapped to some data element.
- Accurate − Definition should be unambiguous.
- Clear and Concise − Definition should be understandable.
Data Structures and Algorithms - Arrays
Array is a container which can hold a fix number of items and these
items should be of the same type. Most of the data structures make use
of arrays to implement their algorithms. Following are the important
terms to understand the concept of Array.
Data Structure and Algorithms - Linked List
A linked list is a sequence of data structures, which are connected together via links.
Linked List is a sequence of links which contains items. Each link contains a connection to another link. Linked list is the second most-used data structure after array. Following are the important terms to understand the concept of Linked List.
Linked List is a sequence of links which contains items. Each link contains a connection to another link. Linked list is the second most-used data structure after array. Following are the important terms to understand the concept of Linked List.
Data Structure - Doubly Linked List
Doubly Linked List is a variation of Linked list in which navigation
is possible in both ways, either forward and backward easily as compared
to Single Linked List. Following are the important terms to understand
the concept of doubly linked list.
Data Structure - Circular Linked List
Circular Linked List is a variation of Linked list in which the first
element points to the last element and the last element points to the
first element. Both Singly Linked List and Doubly Linked List can be
made into a circular linked list.
Data Structure and Algorithms - Stack
A stack is an Abstract Data Type (ADT), commonly used in most
programming languages. It is named stack as it behaves like a real-world
stack, for example – a deck of cards or a pile of plates, etc.
Data Structure - Expression Parsing
The way to write arithmetic expression is known as a notation.
An arithmetic expression can be written in three different but
equivalent notations, i.e., without changing the essence or output of an
expression. These notations are −
Data Structure and Algorithms - Queue
Queue is an abstract data structure, somewhat similar to Stacks.
Unlike stacks, a queue is open at both its ends. One end is always used
to insert data (enqueue) and the other is used to remove data (dequeue).
Queue follows First-In-First-Out methodology, i.e., the data item
stored first will be accessed first.
Data Structure and Algorithms Linear Search
Linear search is a very simple search algorithm. In this type of
search, a sequential search is made over all items one by one. Every
item is checked and if a match is found then that particular item is
returned, otherwise the search continues till the end of the data
collection.
Data Structure and Algorithms Binary Search
Binary search is a fast search algorithm with run-time complexity of
Ο(log n). This search algorithm works on the principle of divide and
conquer. For this algorithm to work properly, the data collection should
be in the sorted form.
Data Structure - Interpolation Search
Interpolation search is an improved variant of binary search. This
search algorithm works on the probing position of the required value.
For this algorithm to work properly, the data collection should be in a
sorted form and equally distributed.
Data Structure and Algorithms - Hash Table
Hash Table is a data structure which stores data in an associative
manner. In a hash table, data is stored in an array format, where each
data value has its own unique index value. Access of data becomes very
fast if we know the index of the desired data.
Data Structure - Sorting Techniques
Sorting refers to arranging data in a particular format. Sorting
algorithm specifies the way to arrange data in a particular order. Most
common orders are in numerical or lexicographical order.
The importance of sorting lies in the fact that data searching can be optimized to a very high level, if data is stored in a sorted manner. Sorting is also used to represent data in more readable formats.
The importance of sorting lies in the fact that data searching can be optimized to a very high level, if data is stored in a sorted manner. Sorting is also used to represent data in more readable formats.
Data Structure - Bubble Sort Algorithm
Bubble sort is a simple sorting algorithm. This sorting algorithm is
comparison-based algorithm in which each pair of adjacent elements is
compared and the elements are swapped if they are not in order. This
algorithm is not suitable for large data sets as its average and worst
case complexity are of Ο(n2) where n is the number of items.
Data Structure and Algorithms Insertion Sort
This is an in-place comparison-based sorting algorithm. Here, a
sub-list is maintained which is always sorted. For example, the lower
part of an array is maintained to be sorted. An element which is to be
'insert'ed in this sorted sub-list, has to find its appropriate place
and then it has to be inserted there. Hence the name, insertion sort.
Data Structure and Algorithms Selection Sort
Selection sort is a simple sorting algorithm. This sorting algorithm
is an in-place comparison-based algorithm in which the list is divided
into two parts, the sorted part at the left end and the unsorted part at
the right end. Initially, the sorted part is empty and the unsorted
part is the entire list.
Data Structures - Merge Sort Algorithm
Merge sort is a sorting technique based on divide and conquer
technique. With worst-case time complexity being Ο(n log n), it is one
of the most respected algorithms.
Merge sort first divides the array into equal halves and then combines them in a sorted manner.
Merge sort first divides the array into equal halves and then combines them in a sorted manner.
Data Structure and Algorithms - Shell Sort
Shell sort is a highly efficient sorting algorithm and is based on
insertion sort algorithm. This algorithm avoids large shifts as in case
of insertion sort, if the smaller value is to the far right and has to
be moved to the far left.
Data Structure and Algorithms - Quick Sort
Quick sort is a highly efficient sorting algorithm and is based on
partitioning of array of data into smaller arrays. A large array is
partitioned into two arrays one of which holds values smaller than the
specified value, say pivot, based on which the partition is made and
another array holds values greater than the pivot value.
Data Structure - Graph Data Structure
A graph is a pictorial representation of a set of objects where some
pairs of objects are connected by links. The interconnected objects are
represented by points termed as vertices, and the links that connect the vertices are called edges.
Data Structure - Depth First Traversal
Depth First Search (DFS) algorithm traverses a graph in a depthward
motion and uses a stack to remember to get the next vertex to start a
search, when a dead end occurs in any iteration.
Data Structure - Breadth First Traversal
Breadth First Search (BFS) algorithm traverses a graph in a
breadthward motion and uses a queue to remember to get the next vertex
to start a search, when a dead end occurs in any iteration.
Data Structure and Algorithms - Tree
Tree represents the nodes connected by edges. We will discuss binary tree or binary search tree specifically.
Binary Tree is a special datastructure used for data storage purposes. A binary tree has a special condition that each node can have a maximum of two children. A binary tree has the benefits of both an ordered array and a linked list as search is as quick as in a sorted array and insertion or deletion operation are as fast as in linked list.
Binary Tree is a special datastructure used for data storage purposes. A binary tree has a special condition that each node can have a maximum of two children. A binary tree has the benefits of both an ordered array and a linked list as search is as quick as in a sorted array and insertion or deletion operation are as fast as in linked list.
Data Structure & Algorithms - Tree Traversal
Traversal is a process to visit all the nodes of a tree and may print
their values too. Because, all nodes are connected via edges (links) we
always start from the root (head) node. That is, we cannot randomly
access a node in a tree. There are three ways which we use to traverse a
tree −
Data Structure - Binary Search Tree
A Binary Search Tree (BST) is a tree in which all the nodes follow the below-mentioned properties −
- The left sub-tree of a node has a key less than or equal to its parent node's key.
- The right sub-tree of a node has a key greater than to its parent node's key.
Data Structure and Algorithms - AVL Trees
What if the input to binary search tree comes in a sorted (ascending or descending) manner? It will then look like this −
Data Structure & Algorithms - Spanning Tree
A spanning tree is a subset of Graph G, which has all the vertices
covered with minimum possible number of edges. Hence, a spanning tree
does not have cycles and it cannot be disconnected..
Heap Data Structures
Heap is a special case of balanced binary tree data structure where
the root-node key is compared with its children and arranged
accordingly. If α has child node β then −
Max-Heap − Where the value of the root node is greater than or equal to either of its children.
Both trees are constructed using the same input and order of arrival.
We are going to derive an algorithm for max heap by inserting one element at a time. At any point of time, heap must maintain its property. While insertion, we also assume that we are inserting a node in an already heapified tree.
Let's understand Max Heap construction by an animated illustration. We consider the same input sample that we used earlier.
key(α) ≥ key(β)
As the value of parent is greater than that of child, this property generates Max Heap. Based on this criteria, a heap can be of two types −For Input → 35 33 42 10 14 19 27 44 26 31Min-Heap − Where the value of the root node is less than or equal to either of its children.

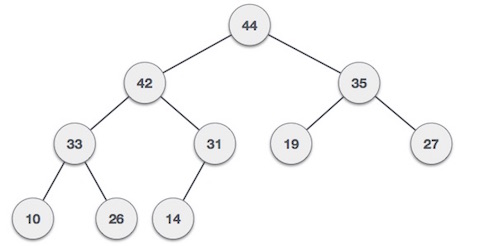
Max Heap Construction Algorithm
We shall use the same example to demonstrate how a Max Heap is created. The procedure to create Min Heap is similar but we go for min values instead of max values.We are going to derive an algorithm for max heap by inserting one element at a time. At any point of time, heap must maintain its property. While insertion, we also assume that we are inserting a node in an already heapified tree.
Step 1 − Create a new node at the end of heap. Step 2 − Assign new value to the node. Step 3 − Compare the value of this child node with its parent. Step 4 − If value of parent is less than child, then swap them. Step 5 − Repeat step 3 & 4 until Heap property holds.Note − In Min Heap construction algorithm, we expect the value of the parent node to be less than that of the child node.
Let's understand Max Heap construction by an animated illustration. We consider the same input sample that we used earlier.
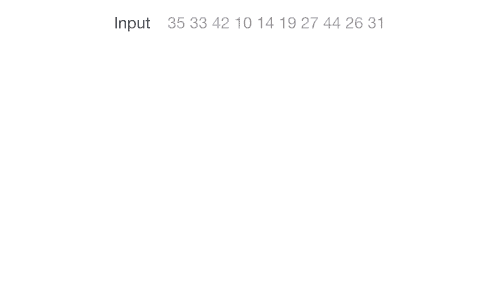
Max Heap Deletion Algorithm
Let us derive an algorithm to delete from max heap. Deletion in Max (or Min) Heap always happens at the root to remove the Maximum (or minimum) value.Data Structure - Recursion Basics
Some computer programming languages allow a module or function to
call itself. This technique is known as recursion. In recursion, a
function α either calls itself directly or calls a function β that in turn calls the original function α. The function α is called recursive function.
Data Structure & Algorithms - Tower of Hanoi
Tower of Hanoi, is a mathematical puzzle which consists of three towers (pegs) and more than one rings is as depicted −
These rings are of different sizes and stacked upon in an ascending
order, i.e. the smaller one sits over the larger one. There are other
variations of the puzzle where the number of disks increase, but the
tower count remains the same.
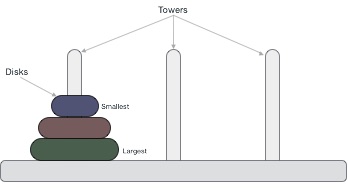
Data Structure & Algorithms Fibonacci Series
Fibonacci series generates the subsequent number by adding two previous numbers. Fibonacci series starts from two numbers − F0 & F1. The initial values of F0 & F1 can be taken 0, 1 or 1, 1 respectively.
Fibonacci series satisfies the following conditions −
Fibonacci series satisfies the following conditions −
DSA Questions & Answers
Data Structures Algorithms Questions and Answers has been designed with a special intention of helping students and professionals preparing for various Certification Exams and Job Interviews.
This section provides a useful collection of sample Interview Questions
and Multiple Choice Questions (MCQs) and their answers with
appropriate explanations.
Data Structures & Algorithms - Quick Guide
Data Structures & Algorithms - Overview
Data Structure is a systematic way to organize data in order to use it efficiently. Following terms are the foundation terms of a data structure.Data Structure - Useful Resources
The following resources contain additional information on Data
Structures and Algorithms. Please use them to get more in-depth
knowledge on this topic.
Discuss Data Structures & Algorithms
Data Structures are the programmatic way of storing data so that data
can be used efficiently. Almost every enterprise application uses
various types of data structures in one or the other way. This tutorial
will give you a great understanding on Data Structures needed to
understand the complexity of enterprise level applications and need of
algorithms, and data structures.
D Programming - Overview
D programming language is an object oriented multi-paradigm system
programming language developed by Walter Bright of Digital Mars. It's
development started in 1999 and was first released in 2001. The major
version of D(1.0) was released in 2007. Currently, we have D2 version of
D.
D - Environment
Try it Option Online
You really do not need to set up your own environment to start learning D programming language. Reason is very simple, we already have set up D Programming environment online, so that you can build and execute all the available examples online at the same time when you are doing your theory work.
D - Basic Syntax
D is quite simple to learn and lets start creating our first D program!
First D Program
Let us write a simple D program. All D files will have extension .d. So put the following source code in a test.d file.import std.stdio;
D - Variables
A variable is nothing but a name given to a storage area that our
programs can manipulate. Each variable in D has a specific type, which
determines the size and layout of the variable's memory; the range of
values that can be stored within that memory; and the set of operations
that can be applied to the variable.
D - Data Types
In the D programming language, data types refer to an extensive
system used for declaring variables or functions of different types. The
type of a variable determines how much space it occupies in storage and
how the bit pattern stored is interpreted.
D - Enums
An enumeration is used for defining named constant values. An enumerated type is declared using the enum keyword.
The enum syntax
The simplest form of an enum definition is the following:enum enum_name { enumeration list }Where,
D - Literals
Constant values that are typed in the program as a part of the source code are called literals.
Literals can be of any of the basic data types and can be divided into Integer Numerals, Floating-Point Numerals, Characters, Strings and Boolean Values.
Literals can be of any of the basic data types and can be divided into Integer Numerals, Floating-Point Numerals, Characters, Strings and Boolean Values.
D - Operators
An operator is a symbol that tells the compiler to perform specific
mathematical or logical manipulations. D language is rich in built-in
operators and provides the following types of operators:
D - Loops
There may be a situation, when you need to execute a block of code
several number of times. In general, statements are executed
sequentially: The first statement in a function is executed first,
followed by the second, and so on.
D - Decisions
Decision making structures require that the programmer specify one or
more conditions to be evaluated or tested by the program, along with a
statement or statements to be executed if the condition is determined to
be true, and optionally, other statements to be executed if the
condition is determined to be false.
D - Functions
Basic function definition
return_type function_name( parameter list ) { body of the function }A basic function definition consists of a function header and a function body. Here are all the parts of a function:
D - Characters
Characters are the building blocks of strings. Any symbol of a
writing system is called a character: letters of alphabets, numerals,
punctuation marks, the space character, etc. Confusingly, the building
blocks of characters themselves are called characters as well.
D - Strings
D provides following two types of string representations:
- Character array.
- Core Language String.
D - Arrays
D programming language provides a data structure, the array,
which stores a fixed-size sequential collection of elements of the same
type. An array is used to store a collection of data, but it is often
more useful to think of an array as a collection of variables of the
same type.
D - Associative Arrays
Associative arrays have an index that is not necessarily an integer,
and can be sparsely populated. The index for an associative array is
called the key, and its type is called the KeyType.
Associative arrays are declared by placing the KeyType within the [ ] of an array declaration. A simple example for associative array is shown below.
Associative arrays are declared by placing the KeyType within the [ ] of an array declaration. A simple example for associative array is shown below.
D - Pointers
D programming pointers are easy and fun to learn. Some D programming
tasks are performed more easily with pointers, and other D programming
tasks, such as dynamic memory allocation, cannot be performed without
them. A simple pointer is shown below.
D - Tuples
Tuples are used for combining multiple values as a single object.
Tuples contains a sequence of elements. The elements can be types,
expressions, or aliases. The number and elements of a tuple are fixed at
compile time and they cannot be changed at run time.
D - Structs
D arrays allow you to define type of variables that can hold several data items of the same kind but structure is another user defined data type available in D programming, which allows you to combine data items of different kinds.
D - Unions
A union is a special data type available in D that enables you
to store different data types in the same memory location. You can
define a union with many members, but only one member can contain a
value at any given time. Unions provide an efficient way of using the
same memory location for multi-purpose.
D - Ranges
Ranges are an abstraction of element access. This abstraction enables
the use of great number of algorithms over great number of container
types. Ranges emphasize how container elements are accessed, as opposed
to how the containers are implemented. Ranges are a very simple concept
that is based on whether a type defines certain sets of member
functions.
D - Aliases
Alias, as the name refers provides an alternate name for existing names. The syntax for alias is shown below.
alias new_name = existing_name;The following is the older syntax, just in case you refer some older format examples. Its is strongly discouraged the use of this.
D - Mixins
Mixins are structs that allows mixing the generated code into the source code. Mixins can be of the following types.
- String Mixins
- Template Mixins
- Mixin name spaces
D - Modules
Modules are the building blocks of D. It is based on a simple
concept. Every source file is a module. Accordingly, the single files
that we have been writing our programs in have all been individual
modules. By default, the name of a module is the same as its filename
without the .d extension.
D - Templates
Templates are the foundation of generic programming, which involves
writing code in a way that is independent of any particular type.
A template is a blueprint or formula for creating a generic class or a function.
A template is a blueprint or formula for creating a generic class or a function.
D - Immutable
We often use variables that are mutable but there can be many
occasions mutability is not required. Immutable variables can be used in
such cases. A few examples are given below where immutable variable can
be used.
D - File I/O
Files are represented by the File struct of the std.stdio module.
A file represents a sequence of bytes, does not matter if it is a text file or binary file. D programming language provides access on high level functions as well as low level (OS level) calls to handle file on your storage devices.
A file represents a sequence of bytes, does not matter if it is a text file or binary file. D programming language provides access on high level functions as well as low level (OS level) calls to handle file on your storage devices.
D - Concurrency
Concurrency is making a program run on more than one thread at a
time. An example of a concurrent program would be a web server
responding more than one client at the same time. Concurrency is easy
with message passing but very difficult to write if they are based on
data sharing.
D - Exception Handling
An exception is a problem that arises during the execution of a
program. A D exception is a response to an exceptional circumstance that
arises while a program is running, such as an attempt to divide by
zero.
Exceptions provide a way to transfer control from one part of a program to another. D exception handling is built upon three keywords: try, catch, and throw.
Exceptions provide a way to transfer control from one part of a program to another. D exception handling is built upon three keywords: try, catch, and throw.
D - Contract Programming
Contract programming in D programming is focused on providing a
simple and understandable means of error handling.Contract programming
in D are implemented by three types of code blocks:
- body block
- in block
- out block
D - Conditional Compilation
Conditional Compilation:
Conditional compilation is the process of selecting which code to compile and which code to not compile similar to the #if / #else / #endif in C and C++. Any statement that is not compiled in still must be syntactically correct. Conditional compilation involves condition checks that are evaluable at compile time.D - Classes & Objects
Classes are the central feature of D programming that supports
object-oriented programming and are often called user-defined types.
A class is used to specify the form of an object and it combines data representation and methods for manipulating that data into one neat package. The data and functions within a class are called members of the class.
A class is used to specify the form of an object and it combines data representation and methods for manipulating that data into one neat package. The data and functions within a class are called members of the class.
D - Inheritance
One of the most important concepts in object-oriented programming is
that of inheritance. Inheritance allows us to define a class in terms of
another class, which makes it easier to create and maintain an
application. This also provides an opportunity to reuse the code
functionality and fast implementation time.
D - Overloading
D allows you to specify more than one definition for a function name or an operator in the same scope, which is called function overloading and operator overloading respectively.
An overloaded declaration is a declaration that had been declared with the same name as a previously declared declaration in the same scope, except that both declarations have different arguments and obviously different definition (implementation).
An overloaded declaration is a declaration that had been declared with the same name as a previously declared declaration in the same scope, except that both declarations have different arguments and obviously different definition (implementation).
D - Encapsulation
All D programs are composed of the following two fundamental elements:
- Program statements (code): This is the part of a program that performs actions and they are called functions.
- Program data: The data is the information of the program which affected by the program functions.
D - Interfaces
An interface is a way of forcing the classes that inherit from it to
have to implement certain functions or variables. Functions must not be
implemented in an interface because they are always implemented in the
classes that inherit from the interface.
D - Abstract Classes
Abstraction refers to the ability to make a class abstract in OOP. An
abstract class is one that cannot be instantiated. All other
functionality of the class still exists, and its fields, methods, and
constructors are all accessed in the same manner. You just cannot create
an instance of the abstract class.
D Programming - Quick Guide
D Programming - Overview
D programming language is an object oriented multi-paradigm system programming language developed by Walter Bright of Digital Mars. It's development started in 1999 and was first released in 2001. The major version of D(1.0) was released in 2007. Currently, we have D2 version of D.D Programming - Useful Resources
The following resources contain additional information on D
Programming. Please use them to get more in-depth knowledge on this
topic.
Discuss D Programming
D programming language is an object oriented multi-paradigm system
programming language. D programming is actually developed by
re-engineering C++ programming language
C Overview
C# is a modern, general-purpose, object-oriented programming language
developed by Microsoft and approved by European Computer Manufacturers
Association (ECMA) and International Standards Organization (ISO).
C Environment
Try it Option Online
We have set up the C# Programming environment online, so that you can compile and execute all the available examples online. It gives you confidence in what you are reading and enables you to verify the programs with different options. Feel free to modify any example and execute it online.
C Program Structure
Before we study basic building blocks of the C# programming language,
let us look at a bare minimum C# program structure so that we can take
it as a reference in upcoming chapters.
C Basic Syntax
C# is an object-oriented programming language. In Object-Oriented
Programming methodology, a program consists of various objects that
interact with each other by means of actions. The actions that an object
may take are called methods. Objects of the same kind are said to have
the same type or, are said to be in the same class.
C# - Data Types
The variables in C#, are categorized into the following types:
- Value types
- Reference types
- Pointer types
C# - Type Conversion
Type conversion is converting one type of data to another type. It is
also known as Type Casting. In C#, type casting has two forms:
C# - Variables
A variable is nothing but a name given to a storage area that our
programs can manipulate. Each variable in C# has a specific type, which
determines the size and layout of the variable's memory the range of
values that can be stored within that memory and the set of operations
that can be applied to the variable.
C# - Constants and Literals
The constants refer to fixed values that the program may not alter
during its execution. These fixed values are also called literals.
Constants can be of any of the basic data types like an integer
constant, a floating constant, a character constant, or a string
literal. There are also enumeration constants as well.
C# - Operators
An operator is a symbol that tells the compiler to perform specific
mathematical or logical manipulations. C# has rich set of built-in
operators and provides the following type of operators:
C# - Decision Making
Decision making structures requires the programmer to specify one or more conditions to be evaluated or tested by the program, along with a statement or statements to be executed if the condition is determined to be true, and optionally, other statements to be executed if the condition is determined to be false.
Following is the general form of a typical decision making structure found in most of the programming languages:
Following is the general form of a typical decision making structure found in most of the programming languages:
C# - Loops
There may be a situation, when you need to execute a block of code several number of times. In general, the statements are executed sequentially: The first statement in a function is executed first, followed by the second, and so on.
C# - Encapsulation
Encapsulation is defined 'as the process of enclosing one or more items within a physical or logical package'. Encapsulation, in object oriented programming methodology, prevents access to implementation details.
C# - Methods
A method is a group of statements that together perform a task. Every
C# program has at least one class with a method named Main.
To use a method, you need to:
To use a method, you need to:
- Define the method
- Call the method
C# - Nullables
C# provides a special data types, the nullable types, to which you can assign normal range of values as well as null values.
For example, you can store any value from -2,147,483,648 to 2,147,483,647 or null in a Nullable<Int32> variable. Similarly, you can assign true, false, or null in a Nullable<bool> variable. Syntax for declaring a nullable type is as follows:
For example, you can store any value from -2,147,483,648 to 2,147,483,647 or null in a Nullable<Int32> variable. Similarly, you can assign true, false, or null in a Nullable<bool> variable. Syntax for declaring a nullable type is as follows:
< data_type> ? <variable_name> = null;The following example demonstrates use of nullable data types:
using System; namespace CalculatorApplication { class NullablesAtShow { static void Main(string[] args) { int? num1 = null; int? num2 = 45; double? num3 = new double?(); double? num4 = 3.14157; bool? boolval = new bool?(); // display the values Console.WriteLine("Nullables at Show: {0}, {1}, {2}, {3}", num1, num2, num3, num4); Console.WriteLine("A Nullable boolean value: {0}", boolval); Console.ReadLine(); } } }When the above code is compiled and executed, it produces the following result:
Nullables at Show: , 45, , 3.14157 A Nullable boolean value:
The Null Coalescing Operator (??)
The null coalescing operator is used with the nullable value types and reference types. It is used for converting an operand to the type of another nullable (or not) value type operand, where an implicit conversion is possible.Sunday, January 29, 2017
C# - Arrays
An array stores a fixed-size sequential collection of elements of the
same type. An array is used to store a collection of data, but it is
often more useful to think of an array as a collection of variables of
the same type stored at contiguous memory locations.
C# - Strings
In C#, you can use strings as array of characters, However, more common practice is to use the string keyword to declare a string variable. The string keyword is an alias for the System.String class.
C# - Structures
In C#, a structure is a value type data type. It helps you to make a
single variable hold related data of various data types. The struct keyword is used for creating a structure.
Structures are used to represent a record. Suppose you want to keep track of your books in a library. You might want to track the following attributes about each book:
Structures are used to represent a record. Suppose you want to keep track of your books in a library. You might want to track the following attributes about each book:
C# - Classes
When you define a class, you define a blueprint for a data type. This
does not actually define any data, but it does define what the class
name means. That is, what an object of the class consists of and what
operations can be performed on that object. Objects are instances of a
class. The methods and variables that constitute a class are called
members of the class.
C# - Inheritance
One of the most important concepts in object-oriented programming is
inheritance. Inheritance allows us to define a class in terms of another
class, which makes it easier to create and maintain an application.
This also provides an opportunity to reuse the code functionality and
speeds up implementation time.
C# - Polymorphism
The word polymorphism means having many forms. In
object-oriented programming paradigm, polymorphism is often expressed as
'one interface, multiple functions'.
Polymorphism can be static or dynamic. In static polymorphism, the response to a function is determined at the compile time. In dynamic polymorphism, it is decided at run-time.
Polymorphism can be static or dynamic. In static polymorphism, the response to a function is determined at the compile time. In dynamic polymorphism, it is decided at run-time.
C# - Operator Overloading
You can redefine or overload most of the built-in operators available
in C#. Thus a programmer can use operators with user-defined types as
well. Overloaded operators are functions with special names the keyword operator
followed by the symbol for the operator being defined. similar to any
other function, an overloaded operator has a return type and a parameter
list.
C# - Interfaces
An interface is defined as a syntactical contract that all the
classes inheriting the interface should follow. The interface defines
the 'what' part of the syntactical contract and the deriving classes define the 'how' part of the syntactical contract.
C# - Namespaces
A namespace is designed for providing a way to keep one set of
names separate from another. The class names declared in one namespace
does not conflict with the same class names declared in another.
C# - Preprocessor Directives
The preprocessor directives give instruction to the compiler to preprocess the information before actual compilation starts.
All preprocessor directives begin with #, and only white-space characters may appear before a preprocessor directive on a line. Preprocessor directives are not statements, so they do not end with a semicolon (;).
All preprocessor directives begin with #, and only white-space characters may appear before a preprocessor directive on a line. Preprocessor directives are not statements, so they do not end with a semicolon (;).
C# - Regular Expressions
A regular expression is a pattern that could be matched
against an input text. The .Net framework provides a regular expression
engine that allows such matching. A pattern consists of one or more
character literals, operators, or constructs.
C# - Exception Handling
An exception is a problem that arises during the execution of a
program. A C# exception is a response to an exceptional circumstance
that arises while a program is running, such as an attempt to divide by
zero.
Exceptions provide a way to transfer control from one part of a program to another. C# exception handling is built upon four keywords: try, catch, finally, and throw.
Exceptions provide a way to transfer control from one part of a program to another. C# exception handling is built upon four keywords: try, catch, finally, and throw.
C# - File I/O
A file is a collection of data stored in a disk with a
specific name and a directory path. When a file is opened for reading or
writing, it becomes a stream.
The stream is basically the sequence of bytes passing through the communication path. There are two main streams: the input stream and the output stream.
The stream is basically the sequence of bytes passing through the communication path. There are two main streams: the input stream and the output stream.
C# - Attributes
An attribute is a declarative tag that is used to convey
information to runtime about the behaviors of various elements like
classes, methods, structures, enumerators, assemblies etc. in your
program. You can add declarative information to a program by using an
attribute. A declarative tag is depicted by square ([ ]) brackets placed
above the element it is used for.
C# - Reflection
Reflection objects are used for obtaining type information at
runtime. The classes that give access to the metadata of a running
program are in the System.Reflection namespace.
C# - Properties
Properties are named members of classes, structures, and interfaces. Member variables or methods in a class or structures are called Fields. Properties are an extension of fields and are accessed using the same syntax. They use accessors through which the values of the private fields can be read, written or manipulated.
C# - Indexers
An indexer allows an object to be indexed such as an array. When you define an indexer for a class, this class behaves similar to a virtual array. You can then access the instance of this class using the array access operator ([ ]).
C# - Delegates
C# delegates are similar to pointers to functions, in C or C++. A delegate is a reference type variable that holds the reference to a method. The reference can be changed at runtime.
Delegates are especially used for implementing events and the call-back methods. All delegates are implicitly derived from the System.Delegate class.
Delegates are especially used for implementing events and the call-back methods. All delegates are implicitly derived from the System.Delegate class.
C# - Events
Events are user actions such as key press, clicks, mouse
movements, etc., or some occurrence such as system generated
notifications. Applications need to respond to events when they occur.
For example, interrupts. Events are used for inter-process
communication.
C# - Collections
Collection classes are specialized classes for data storage and
retrieval. These classes provide support for stacks, queues, lists, and
hash tables. Most collection classes implement the same interfaces.
Collection classes serve various purposes, such as allocating memory dynamically to elements and accessing a list of items on the basis of an index etc.
Collection classes serve various purposes, such as allocating memory dynamically to elements and accessing a list of items on the basis of an index etc.
C# - Generics
Generics allow you to delay the specification of the data type
of programming elements in a class or a method, until it is actually
used in the program. In other words, generics allow you to write a class
or method that can work with any data type.
C# - Anonymous Methods
We discussed that delegates are used to reference any methods that has the same signature as that of the delegate. In other words, you can call a method that can be referenced by a delegate using that delegate object.
C# - Unsafe Codes
C# allows using pointer variables in a function of code block when it is marked by the unsafe modifier. The unsafe code or the unmanaged code is a code block that uses a pointer variable.
Note: To execute the programs mentioned in this chapter at codingground, please set compilation option in Project >> Compile Options >> Compilation Command to
mcs *.cs -out:main.exe -unsafe"
C# - Multithreading
A thread is defined as the execution path of a program. Each
thread defines a unique flow of control. If your application involves
complicated and time consuming operations, then it is often helpful to
set different execution paths or threads, with each thread performing a
particular job.
C# Questions and Answers
C# Questions and Answers has been designed with a special intention of helping students and professionals preparing for various Certification Exams and Job Interviews. This section provides a useful collection of sample Interview Questions and Multiple Choice Questions (MCQs) and their answers with appropriate explanations.
C# - Quick Guide
C# is a modern, general-purpose, object-oriented programming language
developed by Microsoft and approved by European Computer Manufacturers
Association (ECMA) and International Standards Organization (ISO).
C# - Useful Resources
The following resources contain additional information on C#. Please use them to get more in-depth knowledge on this topic.
Discuss C#
C# is a simple, modern, general-purpose, object-oriented programming
language developed by Microsoft within its .NET initiative led by Anders
Hejlsberg. This tutorial will teach you basic C# programming and will
also take you through various advanced concepts related to C#
programming language.
Saturday, January 28, 2017
C Language - Overview
C is a general-purpose, high-level language that was originally
developed by Dennis M. Ritchie to develop the UNIX operating system at
Bell Labs. C was originally first implemented on the DEC PDP-11 computer
in 1972.
C - Environment Setup
Try it Option Online
We have set up the C Programming environment on-line, so that you can compile and execute all the available examples on line. It gives you confidence in what you are reading and enables you to verify the programs with different options. Feel free to modify any example and execute it on-line.
C - Basic Syntax
You have seen the basic structure of a C program, so it will be easy
to understand other basic building blocks of the C programming language.
C - Data Types
Data types in c refer to an extensive system used for declaring
variables or functions of different types. The type of a variable
determines how much space it occupies in storage and how the bit pattern
stored is interpreted.
C - Variables
A variable is nothing but a name given to a storage area that our
programs can manipulate. Each variable in C has a specific type, which
determines the size and layout of the variable's memory; the range of
values that can be stored within that memory; and the set of operations
that can be applied to the variable.
C - Constants & Literals
Constants refer to fixed values that the program may not alter during its execution. These fixed values are also called literals.
Constants can be of any of the basic data types like an integer constant, a floating constant, a character constant, or a string literal. There are enumeration constants as well.
Constants are treated just like regular variables except that their values cannot be modified after their definition.
Constants can be of any of the basic data types like an integer constant, a floating constant, a character constant, or a string literal. There are enumeration constants as well.
Constants are treated just like regular variables except that their values cannot be modified after their definition.
C - Storage Classes
A storage class defines the scope (visibility) and life-time of
variables and/or functions within a C Program. They precede the type
that they modify. We have four different storage classes in a C program −
C - Operators
An operator is a symbol that tells the compiler to perform specific
mathematical or logical functions. C language is rich in built-in
operators and provides the following types of operators −
C - Decision Making
Decision making structures require that the programmer specifies one
or more conditions to be evaluated or tested by the program, along with a
statement or statements to be executed if the condition is determined
to be true, and optionally, other statements to be executed if the
condition is determined to be false.
C - Loops
You may encounter situations, when a block of code needs to be
executed several number of times. In general, statements are executed
sequentially: The first statement in a function is executed first,
followed by the second, and so on.
C - Functions
A function is a group of statements that together perform a task. Every C program has at least one function, which is main(), and all the most trivial programs can define additional functions.
You can divide up your code into separate functions. How you divide up your code among different functions is up to you, but logically the division is such that each function performs a specific task.
You can divide up your code into separate functions. How you divide up your code among different functions is up to you, but logically the division is such that each function performs a specific task.
C - Scope Rules
A scope in any programming is a region of the program where a defined
variable can have its existence and beyond that variable it cannot be
accessed. There are three places where variables can be declared in C
programming language −
C - Arrays
Arrays a kind of data structure that can store a fixed-size
sequential collection of elements of the same type. An array is used to
store a collection of data, but it is often more useful to think of an
array as a collection of variables of the same type.
C - Pointers
Pointers in C are easy and fun to learn. Some C programming tasks are
performed more easily with pointers, and other tasks, such as dynamic
memory allocation, cannot be performed without using pointers. So it
becomes necessary to learn pointers to become a perfect C programmer.
Let's start learning them in simple and easy steps.
C - Strings
Strings are actually one-dimensional array of characters terminated by a null character '\0'. Thus a null-terminated string contains the characters that comprise the string followed by a null.
The following declaration and initialization create a string consisting of the word "Hello". To hold the null character at the end of the array, the size of the character array containing the string is one more than the number of characters in the word "Hello."
The following declaration and initialization create a string consisting of the word "Hello". To hold the null character at the end of the array, the size of the character array containing the string is one more than the number of characters in the word "Hello."
C - Structures
Arrays allow to define type of variables that can hold several data items of the same kind. Similarly structure is another user defined data type available in C that allows to combine data items of different kinds.
C - Unions
A union is a special data type available in C that allows to
store different data types in the same memory location. You can define a
union with many members, but only one member can contain a value at any
given time. Unions provide an efficient way of using the same memory
location for multiple-purpose.
C - Bit Fields
Suppose your C program contains a number of TRUE/FALSE variables grouped in a structure called status, as follows −
struct { unsigned int widthValidated; unsigned int heightValidated; } status;
C - typedef
The C programming language provides a keyword called typedef, which you can use to give a type, a new name. Following is an example to define a term BYTE for one-byte numbers −
typedef unsigned char BYTE;
C - Input & Output
When we say Input, it means to feed some data into a program.
An input can be given in the form of a file or from the command line. C
programming provides a set of built-in functions to read the given input
and feed it to the program as per requirement.
C - File I/O
The last chapter explained the standard input and output devices
handled by C programming language. This chapter cover how C programmers
can create, open, close text or binary files for their data storage.
A file represents a sequence of bytes, regardless of it being a text file or a binary file.
A file represents a sequence of bytes, regardless of it being a text file or a binary file.
C - Preprocessors
The C Preprocessor is not a part of the compiler, but is a
separate step in the compilation process. In simple terms, a C
Preprocessor is just a text substitution tool and it instructs the
compiler to do required pre-processing before the actual compilation.
We'll refer to the C Preprocessor as CPP.
C - Header Files
A header file is a file with extension .h which contains C
function declarations and macro definitions to be shared between several
source files. There are two types of header files: the files that the
programmer writes and the files that comes with your compiler.
C - Type Casting
Type casting is a way to convert a variable from one data type to
another data type. For example, if you want to store a 'long' value into
a simple integer then you can type cast 'long' to 'int'. You can
convert the values from one type to another explicitly using the cast operator as follows −
(type_name) expression
C - Error Handling
As such, C programming does not provide direct support for error
handling but being a system programming language, it provides you access
at lower level in the form of return values. Most of the C or even Unix
function calls return -1 or NULL in case of any error and set an error
code errno. It is set as a global variable and indicates an error
occurred during any function call. You can find various error codes
defined in <error.h> header file.
C - Recursion
Recursion is the process of repeating items in a self-similar way. In
programming languages, if a program allows you to call a function
inside the same function, then it is called a recursive call of the
function.
void recursion() { recursion(); /* function calls itself */
C - Variable Arguments
Sometimes, you may come across a situation, when you want to have a
function, which can take variable number of arguments, i.e., parameters,
instead of predefined number of parameters. The C programming language
provides a solution for this situation and you are allowed to define a
function which can accept variable number of parameters based on your
requirement.
C - Memory Management
This chapter explains dynamic memory management in C. The C
programming language provides several functions for memory allocation
and management. These functions can be found in the <stdlib.h> header file.
C - Command Line Arguments
It is possible to pass some values from the command line to your C programs when they are executed. These values are called command line arguments
and many times they are important for your program especially when you
want to control your program from outside instead of hard coding those
values inside the code.
C Programming Questions and Answers
C Programming Questions and Answers has been designed with a special intention of helping students and professionals preparing for various Certification Exams and Job Interviews.
This section provides a useful collection of sample Interview Questions
and Multiple Choice Questions (MCQs) and their answers with
appropriate explanations.
C - Quick Guide
C - Language Overview
C is a general-purpose, high-level language that was originally developed by Dennis M. Ritchie to develop the UNIX operating system at Bell Labs. C was originally first implemented on the DEC PDP-11 computer in 1972.C - Useful Resources
The following resources contain additional information on C. Please use them to get more in-depth knowledge on this topic.
Discuss C
C is a general-purpose, procedural, imperative computer programming
language developed in 1972 by Dennis M. Ritchie at the Bell Telephone
Laboratories to develop the UNIX operating system. C is the most widely
used computer language. It keeps fluctuating at number one scale of
popularity along with Java programming language, which is also equally
popular and most widely used among modern software programmers.
C++ Library -
Introduction
This data type represents the file stream generally, and has the capabilities of both ofstream and ifstream which means it can create files, write information to files, and read information from files.
C++ Library -
Introduction
iomanip is a library that is used to manipulate the output of C++ program. Using C++, header providing parametric manipulators as shown below −
C++ Library -
Introduction
The C++ standard libraries provide an extensive set of input/output capabilities which we will see in subsequent chapters. This chapter will discuss very basic and most common I/O operations required for C++ programming.Input-Output base classes and types for the IOStream hierarchy of classes as shown below −
C++ Library -
Description
It is used to input-Output forward declarations,this header provides forward declarations for the types of the standard input/output library.
C++ Library -
Description
It is used in standard Input / Output Streams Library.Declaration
Following is the declaration for iosstream function.
C++ Library -
Description
The Istream used for header providing the standard input and combined input/output stream classes.Class templates
C++ Library -
Introduction
It is an output stream objects can write sequences of characters and represent other kinds of data. Specific members are provided to perform these output operations.
C++ Library -
Introduction
It is a string stream for header.Class Templates
Following are the Class Templates for sstram.S.N. | Class Templates | Definition |
---|---|---|
1 | basic_istringstream | It is an input string stream |
2 | basic_ostringstream | It is an output string stream |
3 | basic_stringstream | It is a basic string steam |
4 | basic_stringbuf | It is a string stream buffer |
C++ Library -
Introduction
It is a stream buffer and it is to be used in combination with input/output streams.Class templates
S.N. | Class | Definition |
---|---|---|
1 | basic_streambuf | It is a basic stream buffer |
2 | wstreambuf | It is used in base buffer class in stream |
C++ Library -
Introduction
These are types that encapsulate a value whose access is guaranteed to not cause data races and can be used to synchronize memory accesses among different threads and he atomic library provides components for fine-grained atomic operations allowing for lockless concurrent programming. Each atomic operation is indivisible with regards to any other atomic operation that involves the same object.
C++ Library -
Introduction
It implements the complex class to contain complex numbers in cartesian form and several functions and overloads to operate with them.
C++ Library -
Introduction
It is a standard exception class. All objects thrown by components of the standard library are derived from this class. Therefore, all standard exceptions can be caught by catching this type by reference.
C++ Library -
Introduction
Function objects are objects specifically designed to be used with a syntax similar to that of functions. Instances of std::function can store, copy, and invoke any Callable target -- functions, lambda expressions, bind expressions, or other function objects, as well as pointers to member functions and pointers to data members.
C++ Library -
Introduction
It is a Numeric limits type and it provides information about the properties of arithmetic types (either integral or floating-point) in the specific platform for which the library compiles.
C++ Library -
Introduction
It is a localization library and a set of features that are culture-specific, which can be used by programs to be more portable internationally.
C++ Library -
Introduction
It defines general utilities to manage dynamic memory in header.Allocators
S.N. | Allocator & description |
---|---|
1 | allocator
It is a default allocator. |
Subscribe to:
Posts (Atom)