Highcharts - Overview
Highcharts is a pure JavaScript based charting library meant to enhance web applications by adding interactive charting capability. It supports a wide range of charts. Charts are drawn using SVG in standard browsers like Chrome, Firefox, Safari, Internet Explorer(IE). In legacy IE 6, VML is used to draw the graphics.Features
Following are the salient features of Highcharts library.- Compatability - Works seemlessly on all major browsers and mobile platforms like android and iOS.
- Multitouch Support - Supports multitouch on touch screen based platforms like android and iOS.Ideal for iPhone/iPad and android based smart phones/ tablets.
- Free to Use - Open source and is free to use for non-commercial purpose.
- Lightweight - highcharts.js core library with size nearly 35KB, is extremely lightweight library.
- Simple Configurations - Uses json to define various configuration of the charts and very easy to learn and use.
- Dynamic - Allows to modify chart even after chart generation.
- Multiple axes - Not restricted to x, y axis. Supports multiple axis on the charts.
- Configurable tooltips - Tooltip comes when a user hover over any point on a charts. Highcharts provides tooltip inbuilt formatter or callback formatter to control the tooltip programmatically.
- DateTime support - Handle date time specially. Provides numerous inbuilt controls over date wise categories.
- Export - Export chart to PDF/ PNG/ JPG / SVG format by enabling export feature.
- Print - Print chart using web page.
- Zoomablity - Supports zooming chart to view data more precisely.
- External data - Supports loading data dynamically from server. Provides control over data using callback functions.
- Text Rotation - Supports rotation of labels in any direction.
Supported Chart Types
Highcharts library provides following types of charts:Sr. No. | Chart Type / Description |
---|---|
1 | Line Charts Used to draw line/spline based charts. |
2 | Area Charts Used to draw area wise charts. |
3 | Pie Charts Used to draw pie charts. |
4 | Scatter Charts Used to draw scattered charts. |
5 | Bubble Charts Used to draw bubble based charts. |
6 | Dynamic Charts Used to draw dynamic charts where user can modify charts. |
7 | Combinations Used to draw combinations of variety of charts. |
8 | 3D Charts Used to draw 3D charts. |
9 | Angular Gauges Used to draw speedometer type charts. |
10 | Heat Maps Used to draw heat maps. |
11 | Tree Maps Used to draw tree maps. |
Licence
Highcharts is open source and is free to use for non-commercial purpose. In order to use Highcharts in commercial projects, follow the link: License and PricingHighcharts - Environment Setup
In this chapter we will discuss about how to set up Highcharts library to be used in web application development.Highcharts requires jQuery as a dependency. So first we'll install jQuery library and then HighChart library.
Install jQuery
There are two ways to use jQuery.- Download: Download it locally from jQuery.COM and use it.
- CDN access: You also have access to a CDN. The CDN will give you access around the world to regional data centers that in this case, Google host. This means using CDN moves the responsibility of hosting files from your own servers to a series of external ones. This also offers an advantage that if the visitor to your webpage has already downloaded a copy of jQuery from the same CDN, it won't have to be re-downloaded.
Using Downloaded jQuery
Include the jQuery JavaScript file in the HTML page using following script:<head> <script src="/jquery/jquery.min.js"></script> </head>
Using CDN
We are using the CDN versions of the jQuery library throughout this tutorial.Include the jQuery JavaScript file in the HTML page using following script:
<head> <script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.3/jquery.min.js"></script> </head>
Install Highcharts
There are two ways to use Highcharts.- Download: Download it locally from Highcharts.COM and use it.
- CDN access: You also have access to a CDN. The CDN will give you access around the world to regional data centers that in this case, Highcharts host Code.Highcharts.Com.
Using Downloaded Highcharts
Include the Highcharts JavaScript file in the HTML page using following script:<head> <script src="/highcharts/highcharts.js"></script> </head>
Using CDN
We are using the CDN versions of the Highcharts library throughout this tutorial.Include the Highcharts JavaScript file in the HTML page using following script:
<head> <script src="https://code.highcharts.com/highcharts.js"></script> </head>
Highcharts - Configuration Syntax
In this chapter we'll showcase the comfiguration required to draw a chart using Highcharts API.Step 1: Create HTML Page
Create an HTML page with the jQuery and highchart javascript libraries.HighchartsTestHarness.htm
<html> <head> <title>Highcharts Tutorial</title> <script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.3/jquery.min.js"></script> <script src="https://code.highcharts.com/highcharts.js"></script> </head> <body> <div id="container" style="width: 550px; height: 400px; margin: 0 auto"></div> <script language="JavaScript"> $(document).ready(function() { }); </script> </body> </html>Here container div is used to contain the chart drawn using Highcharts library.
Step 2: Create configurations
Highcharts library uses very simple configurations using json syntax.$('#container').highcharts(json);Here json represents the json data and configuration which HighChart library uses to draw a chart withing container div using highcharts() method. Now we'll configure the various parameter to create the required json string.
title
Configure the title of the chart.var title = { text: 'Monthly Average Temperature' };
subtitle
Configure the subtitle of the chart.var subtitle = { text: 'Source: WorldClimate.com' };
xAxis
Configure the ticker to be displayed on X-Axis.var xAxis = { categories: ['Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun' ,'Jul', 'Aug', 'Sep', 'Oct', 'Nov', 'Dec'] };
yAxis
Configure the title, plot lines to be displayed on Y-Axis.var yAxis = { title: { text: 'Temperature (\xB0C)' }, plotLines: [{ value: 0, width: 1, color: '#808080' }] };
tooltip
Configure the tooltip. Put suffix to be added after value (y-axis).var tooltip = { valueSuffix: '\xB0C' }
legend
Configure the legend to be displayed on the right side of the chart along with other properties.var legend = { layout: 'vertical', align: 'right', verticalAlign: 'middle', borderWidth: 0 };
series
Configure the data to be displayed on the chart. Series is an array where each element of this array represents a single line on the chart.var series = [ { name: 'Tokyo', data: [7.0, 6.9, 9.5, 14.5, 18.2, 21.5, 25.2, 26.5, 23.3, 18.3, 13.9, 9.6] }, { name: 'New York', data: [-0.2, 0.8, 5.7, 11.3, 17.0, 22.0, 24.8, 24.1, 20.1, 14.1, 8.6, 2.5] }, { name: 'Berlin', data: [-0.9, 0.6, 3.5, 8.4, 13.5, 17.0, 18.6, 17.9, 14.3, 9.0, 3.9, 1.0] }, { name: 'London', data: [3.9, 4.2, 5.7, 8.5, 11.9, 15.2, 17.0, 16.6, 14.2, 10.3, 6.6, 4.8] } ];
Step 3: Build the json data
Combine all the configurations.var json = {}; json.title = title; json.subtitle = subtitle; json.xAxis = xAxis; json.yAxis = yAxis; json.tooltip = tooltip; json.legend = legend; json.series = series;
Step 4: Draw the chart
$('#container').highcharts(json);
Example
Following is the complete example:HighchartsTestHarness.htm
<html> <head> <title>Highcharts Tutorial</title> <script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.3/jquery.min.js"></script> <script src="https://code.highcharts.com/highcharts.js"></script> </head> <body> <div id="container" style="width: 550px; height: 400px; margin: 0 auto"></div> <script language="JavaScript"> $(document).ready(function() { var title = { text: 'Monthly Average Temperature' }; var subtitle = { text: 'Source: WorldClimate.com' }; var xAxis = { categories: ['Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun', 'Jul', 'Aug', 'Sep', 'Oct', 'Nov', 'Dec'] }; var yAxis = { title: { text: 'Temperature (\xB0C)' }, plotLines: [{ value: 0, width: 1, color: '#808080' }] }; var tooltip = { valueSuffix: '\xB0C' } var legend = { layout: 'vertical', align: 'right', verticalAlign: 'middle', borderWidth: 0 }; var series = [ { name: 'Tokyo', data: [7.0, 6.9, 9.5, 14.5, 18.2, 21.5, 25.2, 26.5, 23.3, 18.3, 13.9, 9.6] }, { name: 'New York', data: [-0.2, 0.8, 5.7, 11.3, 17.0, 22.0, 24.8, 24.1, 20.1, 14.1, 8.6, 2.5] }, { name: 'Berlin', data: [-0.9, 0.6, 3.5, 8.4, 13.5, 17.0, 18.6, 17.9, 14.3, 9.0, 3.9, 1.0] }, { name: 'London', data: [3.9, 4.2, 5.7, 8.5, 11.9, 15.2, 17.0, 16.6, 14.2, 10.3, 6.6, 4.8] } ]; var json = {}; json.title = title; json.subtitle = subtitle; json.xAxis = xAxis; json.yAxis = yAxis; json.tooltip = tooltip; json.legend = legend; json.series = series; $('#container').highcharts(json); }); </script> </body> </html>
Result
Verify the result.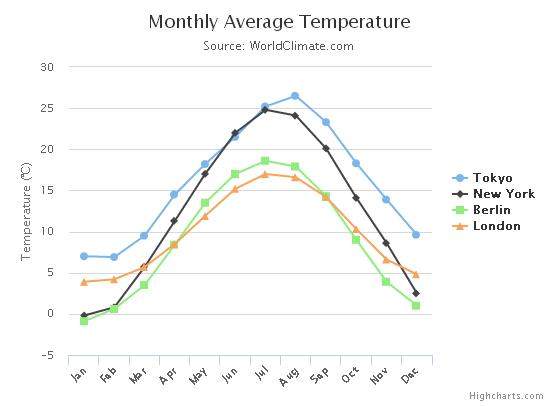
Highcharts - Line Charts
Line charts are used to draw line/spline based charts. In this section we're going to discuss following types of line and spline based charts.Sr. No. | Chart Type / Description |
---|---|
1 | Basic line Basic line chart |
2 | With data labels Chart with data labels |
3 | Ajax loaded data, clickable points Chart drawn after retrieving data from server. |
4 | Time series, zoomable Chart with time series. |
5 | Spline with inverted axes Spline chart having inverted axes. |
6 | Spline with symbols Spline chart using symbols for heat/rain. |
7 | Spline with plot bands Spline chart with plot bands. |
8 | Time data with irregular intervals Chart of a large set of time based data. |
9 | Logarithmic axis Chart depicting the logarithmic axis. |
HighCharts - Area Charts
area charts are used to draw area based charts. In this section we're going to discuss following types of area based charts.Sr. No. | Chart Type / Description |
---|---|
1 | Basic Area Basic area chart |
2 | Area with negative values Area chart having negative values. |
3 | Stacked area Chart having areas stacked over one another. |
4 | Percentage area Chart with data in percentage terms. |
5 | Area with missing points Chart with missing points in the data. |
6 | Inverted axes Area using inverted axes. |
7 | Area-spline Area chart using spline. |
8 | Area range Area chart using ranges. |
9 | Area range and line Area chart using range and line. |
No comments:
Post a Comment