PyGTK - Introduction
PyGTK is a set of wrappers written in Python and C for GTK + GUI library. It is part of the GNOME project. It offers comprehensive tools for building desktop applications in Python. Python bindings for other popular GUI libraries are also available.PyQt is a Python port of QT library. Our PyQt tutorial can be found here. Similarly, wxPython toolkit is Python binding for wxWidgets, another popular cross-platform GUI library. Our wxPython tutorial is available here.
GTK+, or the GIMP Toolkit, is a multi-platform toolkit for creating graphical user interfaces. Offering a complete set of widgets, GTK+ is suitable for projects ranging from small one-off tools to complete application suites.
GTK+ has been designed from the ground up to support a wide range of languages. PyGTK is a Python wrapper for GTK+.
GTK+ is built around the following four libraries −
- Glib − A low-level core library that forms the basis of GTK+. It provides data structure handling for C.
- Pango − A library for layout and rendering of text with an emphasis on internationalization.
- Cairo − A library for 2D graphics with support for multiple output devices (including the X Window System, Win32)
- ATK − A library for a set of interfaces providing accessibility tools such as screen readers, magnifiers, and alternative input devices.
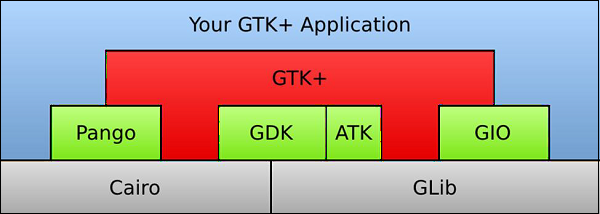
PyGTK is built around GTK + 2.x. In order to build applications for GTK +3, PyGObject bindings are also available.
PyGTK - Environment
PyGTK for Microsoft Windows
The installation of PyGTK for Microsoft Windows involves the following steps −- Step 1 − Install a 32-bit Python interpreter (latest Python 2.7 distribution)
- Step 2 − Download and install GTK+ runtime.
- Step 3 − Download and install GTK+ runtime −http://ftp.gnome.org
- Step 4 − It is also recommended that you download PyCairo and PyGobject modules from the following URLs − http://ftp.gnome.org http://ftp.gnome.org/pub/GNOME
- Step 5 − For convenience, all-in-one installer which handles all of the PyGTK dependencies is also available. Download and install the latest all-in-one installer for Windows from the following URL − http://ftp.gnome.org/pub/GNOME
PyGTK for Linux
PyGTK is included in most Linux distributions (including Debian, Fedora, Ubuntu,RedHat etc); the source code can also be downloaded and compiled from the following URLhttp://ftp.gnome.org/pub/GNOME/sources/pygtk/2.24/
PyGTK - Hello World
Creating a window using PyGTK is very simple. To proceed, we first need to import the gtk module in our code.import gtkThe gtk module contains the gtk.Window class. Its object constructs a toplevel window. We derive a class from gtk.Window.
class PyApp(gtk.Window):Define the constructor and call the show_all() method of the gtk.window class.
def __init__(self): super(PyApp, self).__init__() self.show_all()We now have to declare the object of this class and start an event loop by calling its main() method.
PyApp() gtk.main()It is recommended we add a label “Hello World” in the parent window.
label = gtk.Label("Hello World") self.add(label)The following is a complete code to display “Hello World”−
import gtk class PyApp(gtk.Window): def __init__(self): super(PyApp, self).__init__() self.set_default_size(300,200) self.set_title("Hello World in PyGTK") label = gtk.Label("Hello World") self.add(label) self.show_all() PyApp() gtk.main()The implementation of the above code will yield the following output −

PyGTK - Important Classes
The PyGTK module contains various widgets. gtk.Object class acts as the base class for most of the widgets as well as for some non-widget classes. The toplevel window for desktop applications using PyGTK is provided by gtk.Window class. The following table lists the important widgets and their functions −S.NO | Classes and Description |
---|---|
1 | gtk.Widget This is a gtk.base class for all PyGTK widgets. gtk.Widget provides a common set of methods and signals for the widgets. |
2 | gtk.Window This is a toplevel window that holds one child widget. gtk.Window is a display area decorated with a title bar, and items to allow the user to close, resize and move the window. |
3 | gtk.Button This is a pushbutton widget that issues a signal when clicked. gtk.Button is usually displayed as a pushbutton with a text label and is generally used to attach a callback function. |
4 | gtk.Entry This is a single line text entry widget. |
5 | gtk.Label This widget displays a limited amount of read-only text. |
6 | gtk.ButtonBox This is a base class for widgets that contains multiple buttons. |
7 | gtk.HBox This is a container that organizes its child widgets into a single horizontal row. |
8 | gtk.VBox This is a container that organizes its child widgets into a single column. |
9 | gtk.Fixed This is a container that can place child widgets at fixed positions and with fixed sizes, given in pixels. |
10 | gtk.Layout This provides infinite scrollable area containing child widgets and custom drawing. |
11 | gtk.MenuItem This widget implements the appearance and behavior of menu items. The derived widget subclasses of the gtk.MenuItem are the only valid children of menus. When selected by a user, they can display a popup menu or invoke an associated function or method |
12 | gtk.Menu This is a dropdown menu consisting of a list of MenuItem objects which can be navigated and activated by the user to perform application functions. |
13 | gtk.MenuBar This displays the menu items horizontally in an application window or dialog. |
14 | gtk.ComboBox This widget is used to choose from a list of items. |
15 | gtk.Scale This is a horizontal or vertical slider control to select a numeric value. |
16 | gtk.Scrollbar This displays a horizontal or vertical scrollbar. |
17 | gtk.ProgressBar This is used to display the progress of a long running operation. |
18 | gtk.Dialog This displays a popup window for user information and action. |
19 | gtk.Notebook This widget is a container whose children are overlapping pages that can be switched between using tab labels. |
20 | gtk.Paned This is a base class for widgets with two panes, arranged either horizontally or vertically. Child widgets are added to the panes of the widget. The division between the two children can be adjusted by the user. |
21 | gtk.TextView This widget displays the contents of a TextBuffer object. |
22 | gtk.Toolbar This container holds and manages a set of buttons and widgets in a horizontal or vertical bar. |
23 | gtk.TreeView This widget displays the contents of standard TreeModel (ListStore, TreeStore, TreeModelSort) |
24 | gtk.DrawingArea This widget helps in creating custom user interface elements. gtk.DrawingArea is essentially a blank widget containing a window that you can draw on. |
25 | gtk.Calendar This widget displays a calendar and allows the user to select a date. |
26 | gtk.Viewport This widget displays a portion of a larger widget. |
No comments:
Post a Comment