In this chapter, we will discuss about the integration of BeanEditForm and Grid component
with Hibernate. Hibernate is integrated into the tapestry through the
hibernate module. To enable hibernate module, add tapestry-hibernate
dependency and optionally hsqldb in the pom.xml file. Now, configure hibernate through the hibernate.cfg.xml file placed at the root of the resource folder.
Create an employee class and decorate it with @Entity annotation. Then, add validation annotation for relevant fields and hibernate related annotation @Id and @GeneratedValue for id field. Also, create gender as enum type.
For example, the ListEmployee page can be accessed by a normal URL – (/employee/listemployee) and by the short URL – (/employee/list).
Inject the Hibernate session into the list page using @Inject annotation. Define a property getEmployees in the list page and populate it with employees using injected session object. Complete the code for employee class as shown below.
The complete coding is as follows −
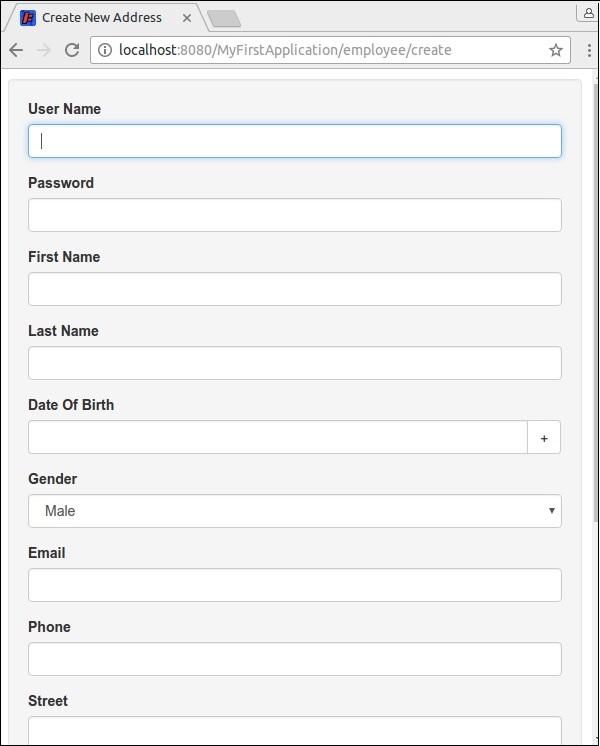
pom.xml (partial)
<dependency> <groupId>org.apache.tapestry</groupId> <artifactId>tapestry-hibernate</artifactId> <version>${tapestry-release-version}</version> </dependency> <dependency> <groupId>org.hsqldb</groupId> <artifactId>hsqldb</artifactId> <version>2.3.2</version> </dependency>
Hibernate.cfg.xml
<!DOCTYPE hibernate-configuration PUBLIC "-//Hibernate/Hibernate Configuration DTD 3.0//EN" "http://hibernate.sourceforge.net/hibernate-configuration-3.0.dtd"> <hibernate-configuration> <session-factory> <property name = "hibernate.connection.driver_class"> org.hsqldb.jdbcDriver </property> <property name = "hibernate.connection.url"> jdbc:hsqldb:./target/work/sampleapp;shutdown = true </property> <property name = "hibernate.dialect"> org.hibernate.dialect.HSQLDialect </property> <property name = "hibernate.connection.username">sa</property> <property name = "hibernate.connection.password"></property> <property name = "hbm2ddl.auto">update</property> <property name = "hibernate.show_sql">true</property> <property name = "hibernate.format_sql">true</property> </session-factory> </hibernate-configuration>Let us see how to create the employee add page using the BeanEditForm component and the employee list page using the Grid component. The persistence layer is handled by Hibernate module.
Create an employee class and decorate it with @Entity annotation. Then, add validation annotation for relevant fields and hibernate related annotation @Id and @GeneratedValue for id field. Also, create gender as enum type.
Employee.java
package com.example.MyFirstApplication.entities; import javax.persistence.Entity; import javax.persistence.GeneratedValue; import javax.persistence.GenerationType; import javax.persistence.Id; import org.apache.tapestry5.beaneditor.NonVisual; import org.apache.tapestry5.beaneditor.Validate; @Entity public class Employee { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) @NonVisual public Long id; @Validate("required") public String firstName; @Validate("required") public String lastName; @Validate("required") public String userName; @Validate("required") public String password; @Validate("required") public String email; public String phone; @Validate("required") public String Street; @Validate("required") public String city; @Validate("required") public String state; @Validate("required,regexp=^\\d{5}(-\\d{4})?$") public String zip; } Gender.java (enum) package com.example.MyFirstApplication.data; public enum Gender { Male, Female }Create the employee list page, ListEmployee.java in the new folder employee under pages and corresponding template file ListEmployee.tml at /src/main/resources/pages/employee folder. Tapestry provides a short URL for sub folders by removing repeated data.
For example, the ListEmployee page can be accessed by a normal URL – (/employee/listemployee) and by the short URL – (/employee/list).
Inject the Hibernate session into the list page using @Inject annotation. Define a property getEmployees in the list page and populate it with employees using injected session object. Complete the code for employee class as shown below.
ListEmployee.java
package com.example.MyFirstApplication.pages.employee; import java.util.List; import org.apache.tapestry5.annotations.Import; import org.apache.tapestry5.ioc.annotations.Inject; import org.hibernate.Session; import com.example.MyFirstApplication.entities.Employee; import org.apache.tapestry5.annotations.Import; @Import(stylesheet="context:mybootstrap/css/bootstrap.css") public class ListEmployee { @Inject private Session session; public List<Employee> getEmployees() { return session.createCriteria(Employee.class).list(); } }Create the template file for ListEmployee class. The template will have two main components, which are −
- PageLink − Create employee link page.
- Grid − Used to render the employee details. The grid component has sources attributes to inject employee list and include attributes to include the fields to be rendered.
<html t:type = "simplelayout" title = "List Employee" xmlns:t = "http://tapestry.apache.org/schema/tapestry_5_4.xsd"> <h1>Employees</h1> <ul> <li><t:pagelink page = "employee/create">Create new employee</t:pagelink></li> </ul> <t:grid source = "employees" include = "userName,firstName,lastName,gender,dateOfBirth,phone,city,state"/> </html>Create employee creation template file and include BeanEditForm component. The component has the following attributes −
- object − Includes source.
- reorder − Defines the order of the fields to be rendered.
- submitlabel − The message of the form submission button
<html t:type = "simplelayout" title = "Create New Address" xmlns:t = "http://tapestry.apache.org/schema/tapestry_5_4.xsd"> <t:beaneditform object = "employee" submitlabel = "message:submit-label" reorder = "userName,password,firstName,lastName, dateOfBirth,gender,email,phone,s treet,city,state,zip" /> </html>Create employee creation class and include session, employee property, list page (navigation link) and define the OnSuccess event (place to update the data) of the component. The session data is persisted into the database using the hibernate session.
The complete coding is as follows −
package com.example.MyFirstApplication.pages.employee; import com.example.MyFirstApplication.entities.Employee; import com.example.MyFirstApplication.pages.employee.ListEmployee; import org.apache.tapestry5.annotations.InjectPage; import org.apache.tapestry5.annotations.Property; import org.apache.tapestry5.hibernate.annotations.CommitAfter; import org.apache.tapestry5.ioc.annotations.Inject; import org.hibernate.Session; public class CreateEmployee { @Property private Employee employee; @Inject private Session session; @InjectPage private ListEmployee listPage; @CommitAfter Object onSuccess() { session.persist(employee); return listPage; } }Add the CreateEmployee.properties file and include the message to be used in form validations. The complete code is as follows −
zip-regexp=^\\d{5}(-\\d{4})?$ zip-regexp-message = Zip Codes are five or nine digits. Example: 02134 or 901251655. submit-label = Create EmployeeThe screenshot of the employee creation page and listing page are shown below −
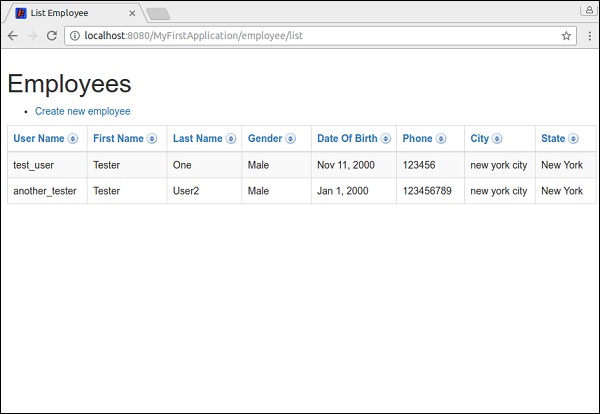
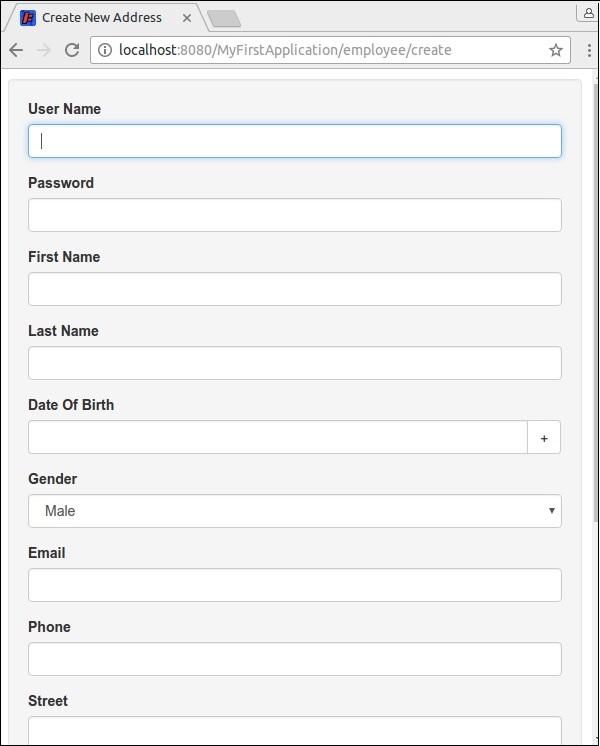
No comments:
Post a Comment