Let us now learn how to remove pages from a PDF document.
While specifying the index for the pages in a PDF document, keep in mind that indexing of these pages starts from zero, i.e., if you want to delete the 1st page then the index value needs to be 0.
This example demonstrates how to remove pages from an existing PDF
document. Here, we will load the above specified PDF document named sample.pdf, remove a page from it, and save it in the path C:/PdfBox_Examples/. Save this code in a file with name Removing_pages.java.
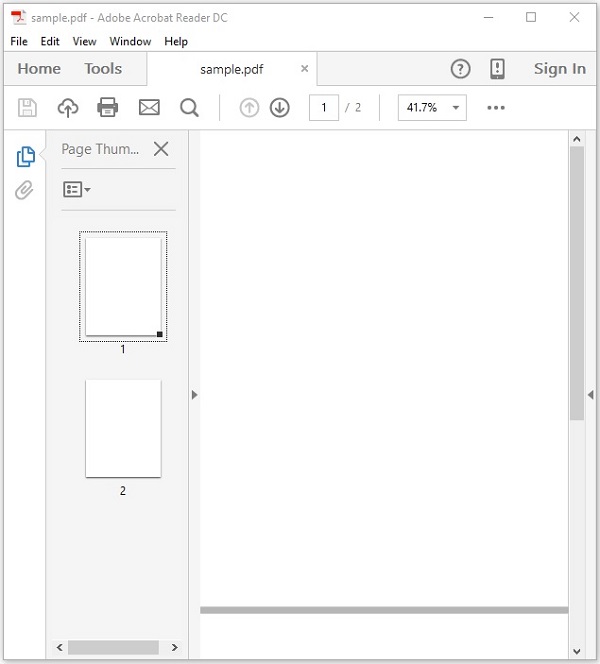
Removing Pages from an Existing Document
You can remove a page from an existing PDF document using the removePage() method of the PDDocument class.Step 1: Loading an Existing PDF Document
Load an existing PDF document using the static method load() of the PDDocument class. This method accepts a file object as a parameter, since this is a static method you can invoke it using class name as shown below.File file = new File("path of the document") PDDocument.load(file);
Step 2: Listing the Number of Pages
You can list the number of pages that exists in the PDF document using the getNumberOfPages() method as shown below.int noOfPages= document.getNumberOfPages(); System.out.print(noOfPages);
Step 3: Removing the Page
You can remove a page from the PDF document using the removePage() method of the PDDocument class. To this method, you need to pass the index of the page that is to be deleted.While specifying the index for the pages in a PDF document, keep in mind that indexing of these pages starts from zero, i.e., if you want to delete the 1st page then the index value needs to be 0.
document.removePage(2);
Step 4: Saving the Document
After removing the page, save the PDF document using the save() method of the PDDocument class as shown in the following code block.document.save("Path");
Step 5: Closing the Document
Finally, close the document using the close() method of the PDDocument class as shown below.document.close();
Example
Suppose, we have a PDF document with name sample.pdf and it contains three empty pages as shown below.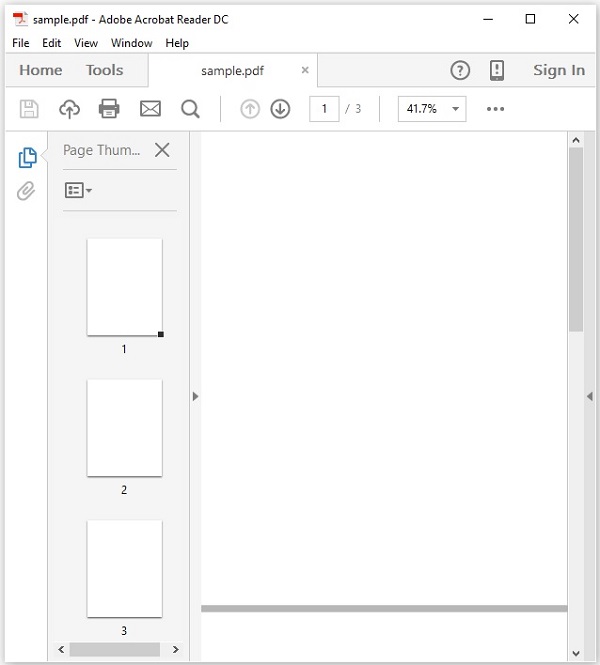
import java.io.File; import java.io.IOException; import org.apache.pdfbox.pdmodel.PDDocument; public class RemovingPages { public static void main(String args[]) throws IOException { //Loading an existing document File file = new File("C:/PdfBox_Examples/sample.pdf"); PDDocument document = PDDocument.load(file); //Listing the number of existing pages int noOfPages= document.getNumberOfPages(); System.out.print(noOfPages); //Removing the pages document.removePage(2); System.out.println("page removed"); //Saving the document document.save("C:/PdfBox_Examples/sample.pdf"); //Closing the document document.close(); } }Compile and execute the saved Java file from the command prompt using the following commands.
javac RemovingPages.java java RemovingPagesUpon execution, the above program creates a PDF document with blank pages displaying the following message.
3 page removedIf you verify the specified path, you can find that the required page was deleted and only two pages remained in the document as shown below.
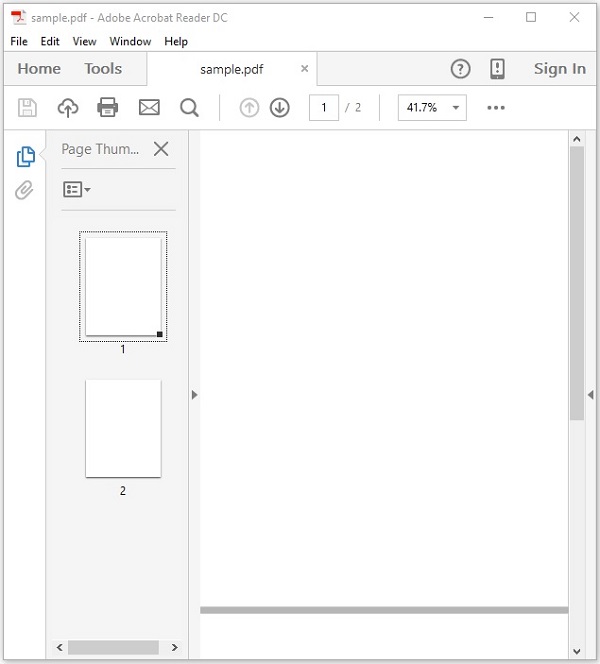
No comments:
Post a Comment