In the previous chapter, we have seen how to split a given PDF
document into multiple documents. Let us now learn how to merge multiple
PDF documents as a single document.
Following are the steps to merge multiple PDF documents.
This example demonstrates how to merge the above PDF documents. Here, we will merge the PDF documents named sample1.pdf and sample2.pdf in to a single PDF document merged.pdf. Save this code in a file with name MergePDFs.java.
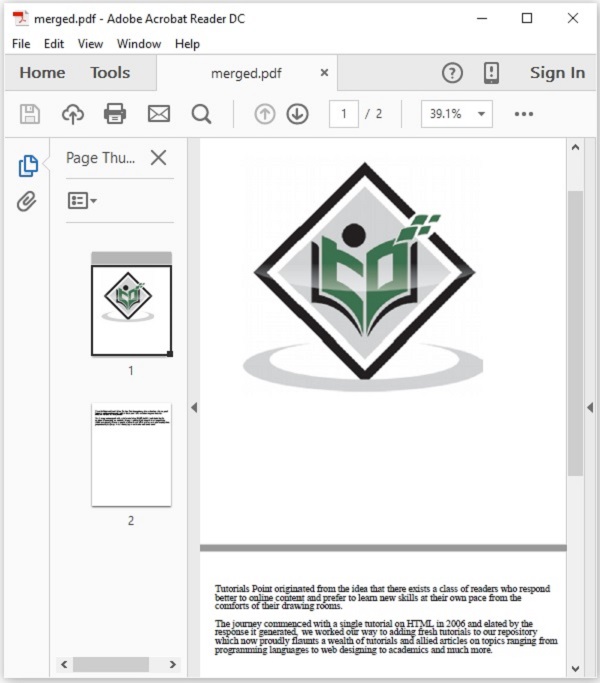
Merging Multiple PDF Documents
You can merge multiple PDF documents into a single PDF document using the class named PDFMergerUtility class, this class provides methods to merge two or more PDF documents in to a single PDF document.Following are the steps to merge multiple PDF documents.
Step 1: Loading an Existing PDF Document
Load an existing PDF document using the static method load() of the PDDocument class. This method accepts a file object as a parameter, since this is a static method you can invoke it using class name as shown below.File file = new File("path of the document") PDDocument document = PDDocument.load(file);
Step 2: Instantiating the PDFMergerUtility class
instantiate the merge utility class as shown below.PDFMergerUtility PDFmerger = new PDFMergerUtility();
Step 3: Setting the destination file
Set the destination files using the setDestinationFileName() method as shown below.PDFmerger.setDestinationFileName("C:/PdfBox_Examples/data1/merged.pdf");
Step 4: Setting the source files
Set the source files using the addSource() method as shown below.PDFmerger.addSource(file1);
Step 5: Merging the documents
Mergr the documents using the mergeDocuments() method of the PDFmerger class as shown below.PDFmerger.addSource(file1);
Step 6: Closing the Document
Finally close the document using close() method of PDDocument class as shown below.document.close();
Example
Suppose, we have two PDF documents — sample1.pdf and sample2.pdf, in the path C:\PdfBox_Examples\ as shown below.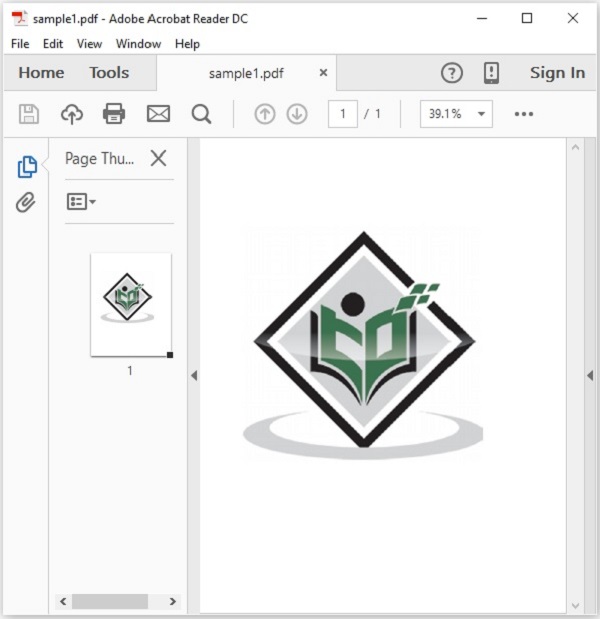
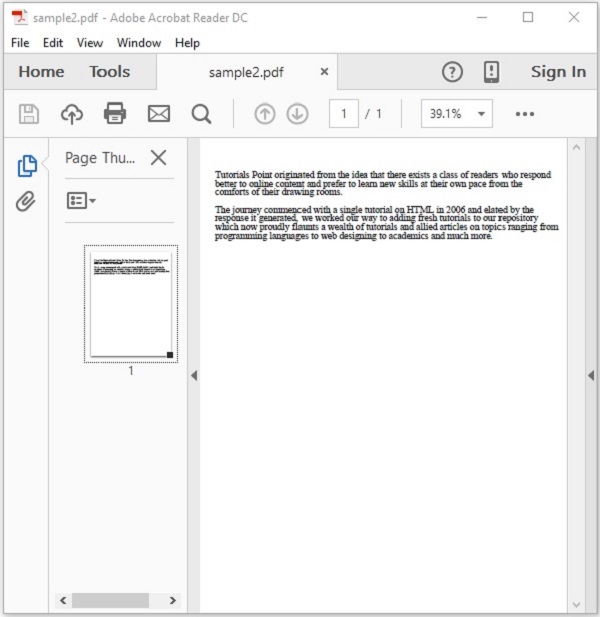
import org.apache.pdfbox.multipdf.PDFMergerUtility; import org.apache.pdfbox.pdmodel.PDDocument; import java.io.File; import java.io.IOException; public class MergePDFs { public static void main(String[] args) throws IOException { //Loading an existing PDF document File file1 = new File("C:/PdfBox_Examples/sample1.pdf"); PDDocument doc1 = PDDocument.load(file1); File file2 = new File("C:/PdfBox_Examples/sample2.pdf"); PDDocument doc2 = PDDocument.load(file2); //Instantiating PDFMergerUtility class PDFMergerUtility PDFmerger = new PDFMergerUtility(); //Setting the destination file PDFmerger.setDestinationFileName("C:/PdfBox_Examples/merged.pdf"); //adding the source files PDFmerger.addSource(file1); PDFmerger.addSource(file2); //Merging the two documents PDFmerger.mergeDocuments(); System.out.println("Documents merged"); //Closing the documents doc1.close(); doc2.close(); } }Compile and execute the saved Java file from the command prompt using the following commands.
javac MergePDFs.java java MergePDFsUpon execution, the above program encrypts the given PDF document displaying the following message.
Documents mergedIf you verify the given path, you can observe that a PDF document with name merged.pdf is created and this contains the pages of both the source documents as shown below.
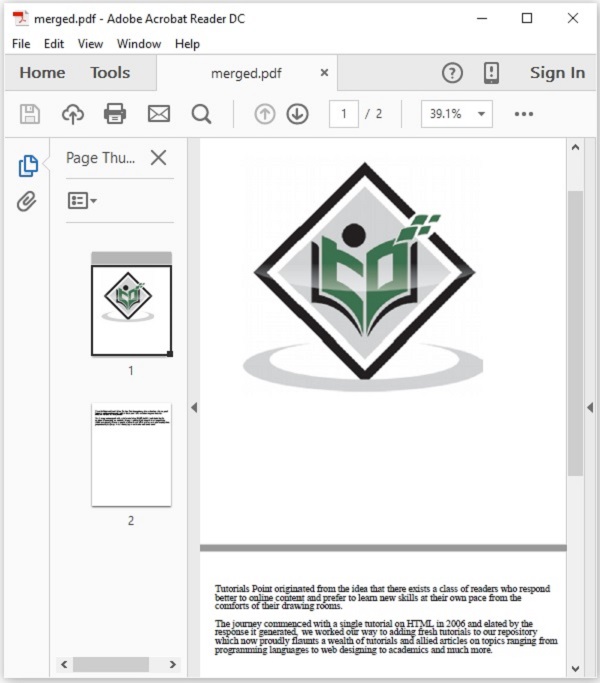
No comments:
Post a Comment