JSF provides a rich expression language. We can write normal
operations using #{operation-expression} notation. Some of the
advantages of JSF Expression languages are following.
- Can reference bean properties where bean can be a object stored in request, session or application scope or is a managed bean.
- Provides easy access to elements of a collection which can be a list, map or an array.
- Provides easy access to predefined objects such as request.
- Arithmetic, logical, relational operations can be done using expression language.
- Automatic type conversion.
- Shows missing values as empty strings instead of NullPointerException.
Example Application
Let us create a test JSF application to test expression language.
Step | Description |
1 | Create a project with a name helloworld under a package com.tutorialspoint.test as explained in the JSF - First Application chapter. |
8 | Modify UserData.java under package com.tutorialspoint.test as explained below. |
9 | Modify home.xhtml as explained below. Keep rest of the files unchanged. |
10 | Compile and run the application to make sure business logic is working as per the requirements. |
11 | Finally, build the application in the form of war file and deploy it in Apache Tomcat Webserver. |
12 | Launch your web application using appropriate URL as explained below in the last step. |
UserData.java
package com.tutorialspoint.test;
import java.io.Serializable;
import java.util.Date;
import javax.faces.bean.ManagedBean;
import javax.faces.bean.SessionScoped;
@ManagedBean(name = "userData", eager = true)
@SessionScoped
public class UserData implements Serializable {
private static final long serialVersionUID = 1L;
private Date createTime = new Date();
private String message = "Hello World!";
public Date getCreateTime() {
return(createTime);
}
public String getMessage() {
return(message);
}
}
home.xhtml
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN"
"http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:f="http://java.sun.com/jsf/core"
xmlns:h="http://java.sun.com/jsf/html">
<h:head>
<title>JSF Tutorial!</title>
</h:head>
<h2>Expression Language Example</h2>
Creation time:
<h:outputText value="#{userData.createTime}"/>
<br/><br/>Message:
<h:outputText value="#{userData.message}"/>
</h:body>
</html>
Once you are ready with all the changes done, let us compile and run
the application as we did in JSF - First Application chapter. If
everything is fine with your application, this will produce following
result:
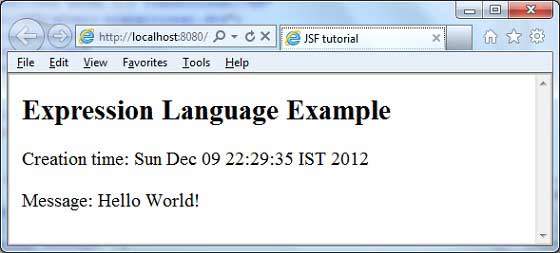
No comments:
Post a Comment