After associating the buffers with the shaders, the final step is to
draw the required primitives. WebGL provides two methods namely, drawArrays() and drawElements() to draw models.
Suppose you want to draw contiguous triangles, then you have to pass
the next three vertices in order in the vertex buffer and mention the
number of elements to be rendered as 6.
If you want to draw contagious triangles using drawElements() method, simply add the other vertices and mention the indices for the remaining vertices.
Take a look at the following example. Here we are passing the RGBA value of gray color.
drawArrays()
drawArrays() is the method which is used to draw models using vertices. Here is its syntax −void drawArrays(enum mode, int first, long count)This method takes the following three parameters −
- mode − In WebGL, models are drawn using primitive types. Using mode, programmers have to choose one of the primitive types provided by WebGL. The possible values for this option are − gl.POINTS, gl.LINE_STRIP, gl.LINE_LOOP, gl.LINES, gl.TRIANGLE_STRIP, gl.TRIANGLE_FAN, and gl.TRIANGLES.
- first − This option specifies the starting element in the enabled arrays. It cannot be a negative value.
- count − This option specifies the number of elements to be rendered.
Example
If you want to draw a single triangle using drawArray() method, then you have to pass three vertices and call the drawArrays() method, as shown below.var vertices = [-0.5,-0.5, -0.25,0.5, 0.0,-0.5,]; gl.drawArrays(gl.TRIANGLES, 0, 3);It will produce a triangle as shown below.

var vertices = [-0.5,-0.5, -0.25,0.5, 0.0,-0.5, 0.0,-0.5, 0.25,0.5, 0.5,-0.5,]; gl.drawArrays(gl.TRIANGLES, 0, 6);It will produce a contiguous triangle as shown below.
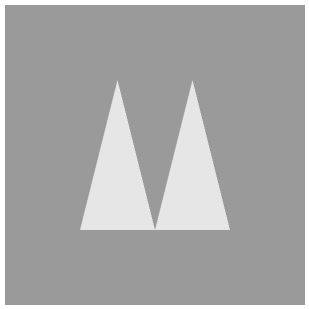
drawElements()
drawElements() is the method that is used to draw models using vertices and indices. Its syntax is as follows −void drawElements(enum mode, long count, enum type, long offset)This method takes the following four parameters −
- mode − WebGL models are drawn using primitive types. Using mode, programmers have to choose one of the primitive types provided by WebGL. The list of possible values for this option are − gl.POINTS, gl.LINE_STRIP, gl.LINE_LOOP, gl.LINES, gl.TRIANGLE_STRIP, gl.TRIANGLE_FAN, and gl.TRIANGLES.
- count − This option specifies the number of elements to be rendered.
- type − This option specifies the data type of the indices which must be UNSIGNED_BYTE or UNSIGNED_SHORT.
- offset − This option specifies the starting point for rendering. It is usually the first element (0).
Example
If you want to draw a single triangle using indices, you need to pass the indices along with vertices and call the drawElements() method as shown below.var vertices = [ -0.5,-0.5,0.0, -0.25,0.5,0.0, 0.0,-0.5,0.0 ]; var indices = [0,1,2]; gl.drawElements(gl.TRIANGLES, indices.length, gl.UNSIGNED_SHORT,0);It will produce the following output −

var vertices = [ -0.5,-0.5,0.0, -0.25,0.5,0.0, 0.0,-0.5,0.0, 0.25,0.5,0.0, 0.5,-0.5,0.0 ]; var indices = [0,1,2,2,3,4]; gl.drawElements(gl.TRIANGLES, indices.length, gl.UNSIGNED_SHORT,0);It will produce the following output −
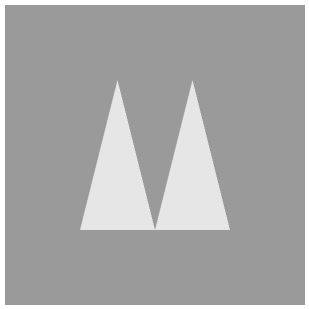
Required Operations
Before drawing a primitive, you need to perform a few operations, which are explained below.Clear the Canvas
First of all, you should clear the canvas, using clearColor() method. You can pass the RGBA values of a desired color as parameter to this method. Then WebGL clears the canvas and fills it with the specified color. Therefore, you can use this method for setting the background color.Take a look at the following example. Here we are passing the RGBA value of gray color.
gl.clearColor(0.5, 0.5, .5, 1);
Enable Depth Test
Enable the depth test using the enable() method, as shown below.gl.enable(gl.DEPTH_TEST);
Clear the Color Buffer Bit
Clear the color as well as the depth buffer by using the clear() method, as shown below.gl.clear(gl.COLOR_BUFFER_BIT | gl.DEPTH_BUFFER_BIT);
Set the View Port
The view port represents a rectangular viewable area that contains the rendering results of the drawing buffer. You can set the dimensions of the view port using viewport() method. In the following code, the view port dimensions are set to the canvas dimensions.gl.viewport(0,0,canvas.width,canvas.height);
No comments:
Post a Comment