In JavaFX, we can develop GUI applications, web applications and
graphical applications. In such applications, whenever a user interacts
with the application (nodes), an event is said to have been occurred.
For example, clicking on a button, moving the mouse, entering a character through keyboard, selecting an item from list, scrolling the page are the activities that causes an event to happen.
An instance of any of its subclass is an event. JavaFX provides a wide variety of events. Some of them are are listed below.
JavaFX provides handlers and filters to handle events. In JavaFX every event has −
If you click on the play button, the source will be the mouse, the
target node will be the play button and the type of the event generated
is the mouse click.
Following is the event dispatch chain for the event generated, when we click on the play button in the above scenario.
As mentioned above, during the event, processing is a filter that is executed and during the event bubbling phase, a handler is executed. All the handlers and filters implement the interface EventHandler of the package javafx.event.
Save this code in a file with name EventHandlersExample.java.
Here, if you type a letter in the text field, the 3D box starts
rotating along the x axis. If you click on the box again the rotation
stops.
Most of these methods exist in the classes like Node, Scene, Window, etc., and they are available to all their sub classes.
For example, to add a mouse event listener to a button, you can use the convenience method setOnMouseClicked() as shown below.
Save this code in a file with the name ConvinienceMethodsExample.java.
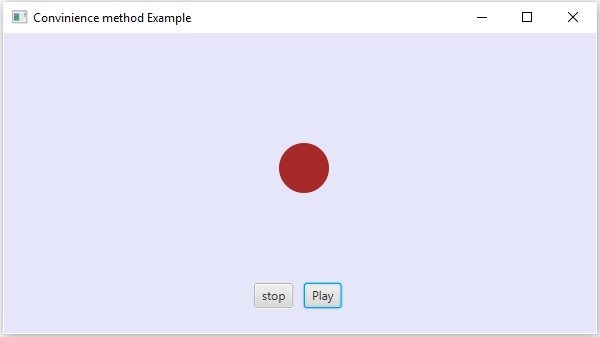
For example, clicking on a button, moving the mouse, entering a character through keyboard, selecting an item from list, scrolling the page are the activities that causes an event to happen.
Types of Events
The events can be broadly classified into the following two categories −- Foreground Events − Those events which require the direct interaction of a user. They are generated as consequences of a person interacting with the graphical components in a Graphical User Interface. For example, clicking on a button, moving the mouse, entering a character through keyboard, selecting an item from list, scrolling the page, etc.
- Background Events − Those events that require the interaction of end user are known as background events. The operating system interruptions, hardware or software failure, timer expiry, operation completion are the example of background events.
Events in JavaFX
JavaFX provides support to handle a wide varieties of events. The class named Event of the package javafx.event is the base class for an event.An instance of any of its subclass is an event. JavaFX provides a wide variety of events. Some of them are are listed below.
- Mouse Event − This is an input event that occurs when a mouse is clicked. It is represented by the class named MouseEvent. It includes actions like mouse clicked, mouse pressed, mouse released, mouse moved, mouse entered target, mouse exited target, etc.
- Key Event − This is an input event that indicates the key stroke occurred on a node. It is represented by the class named KeyEvent. This event includes actions like key pressed, key released and key typed.
- Drag Event − This is an input event which occurs when the mouse is dragged. It is represented by the class named DragEvent. It includes actions like drag entered, drag dropped, drag entered target, drag exited target, drag over, etc.
- Window Event − This is an event related to window showing/hiding actions. It is represented by the class named WindowEvent. It includes actions like window hiding, window shown, window hidden, window showing, etc.
Event Handling
Event Handling is the mechanism that controls the event and decides what should happen, if an event occurs. This mechanism has the code which is known as an event handler that is executed when an event occurs.JavaFX provides handlers and filters to handle events. In JavaFX every event has −
- Target − The node on which an event occurred. A target can be a window, scene, and a node.
- Source − The source from which the event is generated will be the source of the event. In the above scenario, mouse is the source of the event.
- Type − Type of the occurred event; in case of mouse event – mouse pressed, mouse released are the type of events.
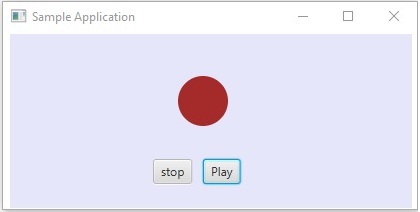
Phases of Event Handling in JavaFX
Whenever an event is generated, JavaFX undergoes the following phases.Route Construction
Whenever an event is generated, the default/initial route of the event is determined by construction of an Event Dispatch chain. It is the path from the stage to the source Node.Following is the event dispatch chain for the event generated, when we click on the play button in the above scenario.
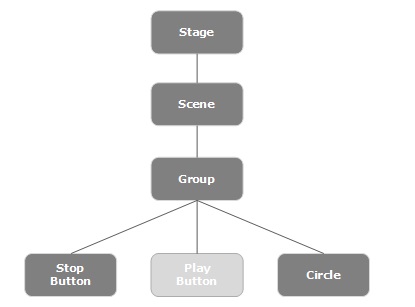
Event Capturing Phase
After the construction of the event dispatch chain, the root node of the application dispatches the event. This event travels to all nodes in the dispatch chain (from top to bottom). If any of these nodes has a filter registered for the generated event, it will be executed. If none of the nodes in the dispatch chain has a filter for the event generated, then it is passed to the target node and finally the target node processes the event.Event Bubbling Phase
In the event bubbling phase, the event is travelled from the target node to the stage node (bottom to top). If any of the nodes in the event dispatch chain has a handler registered for the generated event, it will be executed. If none of these nodes have handlers to handle the event, then the event reaches the root node and finally the process will be completed.Event Handlers and Filters
Event filters and handlers are those which contains application logic to process an event. A node can register to more than one handler/filter. In case of parent–child nodes, you can provide a common filter/handler to the parents, which is processed as default for all the child nodes.As mentioned above, during the event, processing is a filter that is executed and during the event bubbling phase, a handler is executed. All the handlers and filters implement the interface EventHandler of the package javafx.event.
Adding and Removing Event Filter
To add an event filter to a node, you need to register this filter using the method addEventFilter() of the Node class.//Creating the mouse event handler EventHandler<MouseEvent> eventHandler = new EventHandler<MouseEvent>() { @Override public void handle(MouseEvent e) { System.out.println("Hello World"); circle.setFill(Color.DARKSLATEBLUE); } }; //Adding event Filter Circle.addEventFilter(MouseEvent.MOUSE_CLICKED, eventHandler);In the same way, you can remove a filter using the method removeEventFilter() as shown below −
circle.removeEventFilter(MouseEvent.MOUSE_CLICKED, eventHandler);
Event Handling Example
Following is an example demonstrating the event handling in JavaFX using the event filters. Save this code in a file with name EventFiltersExample.java.import javafx.application.Application; import static javafx.application.Application.launch; import javafx.event.EventHandler; import javafx.scene.Group; import javafx.scene.Scene; import javafx.scene.input.MouseEvent; import javafx.scene.paint.Color; import javafx.scene.shape.Circle; import javafx.scene.text.Font; import javafx.scene.text.FontWeight; import javafx.scene.text.Text; import javafx.stage.Stage; public class EventFiltersExample extends Application { @Override public void start(Stage stage) { //Drawing a Circle Circle circle = new Circle(); //Setting the position of the circle circle.setCenterX(300.0f); circle.setCenterY(135.0f); //Setting the radius of the circle circle.setRadius(25.0f); //Setting the color of the circle circle.setFill(Color.BROWN); //Setting the stroke width of the circle circle.setStrokeWidth(20); //Setting the text Text text = new Text("Click on the circle to change its color"); //Setting the font of the text text.setFont(Font.font(null, FontWeight.BOLD, 15)); //Setting the color of the text text.setFill(Color.CRIMSON); //setting the position of the text text.setX(150); text.setY(50); //Creating the mouse event handler EventHandler<MouseEvent> eventHandler = new EventHandler<MouseEvent>() { @Override public void handle(MouseEvent e) { System.out.println("Hello World"); circle.setFill(Color.DARKSLATEBLUE); } }; //Registering the event filter circle.addEventFilter(MouseEvent.MOUSE_CLICKED, eventHandler); //Creating a Group object Group root = new Group(circle, text); //Creating a scene object Scene scene = new Scene(root, 600, 300); //Setting the fill color to the scene scene.setFill(Color.LAVENDER); //Setting title to the Stage stage.setTitle("Event Filters Example"); //Adding scene to the stage stage.setScene(scene); //Displaying the contents of the stage stage.show(); } public static void main(String args[]){ launch(args); } }Compile and execute the saved java file from the command prompt using the following commands.
javac EventFiltersExample.java java EventFiltersExampleOn executing, the above program generates a JavaFX window as shown below.
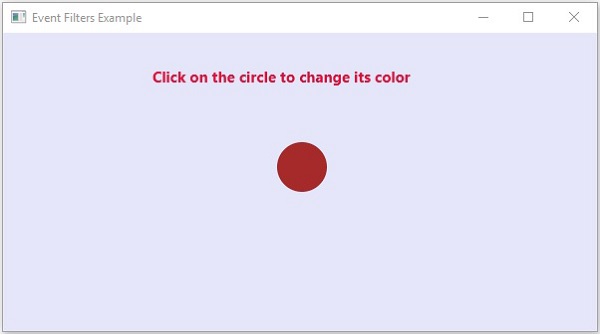
Adding and Removing Event Handlers
To add an event handler to a node, you need to register this handler using the method addEventHandler() of the Node class as shown below.//Creating the mouse event handler EventHandler<javafx.scene.input.MouseEvent> eventHandler = new EventHandler<javafx.scene.input.MouseEvent>() { @Override public void handle(javafx.scene.input.MouseEvent e) { System.out.println("Hello World"); circle.setFill(Color.DARKSLATEBLUE); } }; //Adding the event handler circle.addEventHandler(javafx.scene.input.MouseEvent.MOUSE_CLICKED, eventHandler);In the same way, you can remove an event handler using the method removeEventHandler() as shown below −
circle.removeEventHandler(MouseEvent.MOUSE_CLICKED, eventHandler);
Example
The following program is an example demonstrating the event handling in JavaFX using the event handlers.Save this code in a file with name EventHandlersExample.java.
import javafx.animation.RotateTransition; import javafx.application.Application; import javafx.event.EventHandler; import javafx.scene.Group; import javafx.scene.PerspectiveCamera; import javafx.scene.Scene; import javafx.scene.control.TextField; import javafx.scene.input.KeyEvent; import javafx.scene.paint.Color; import javafx.scene.paint.PhongMaterial; import javafx.scene.shape.Box; import javafx.scene.text.Font; import javafx.scene.text.FontWeight; import javafx.scene.text.Text; import javafx.scene.transform.Rotate; import javafx.stage.Stage; import javafx.util.Duration; public class EventHandlersExample extends Application { @Override public void start(Stage stage) { //Drawing a Box Box box = new Box(); //Setting the properties of the Box box.setWidth(150.0); box.setHeight(150.0); box.setDepth(100.0); //Setting the position of the box box.setTranslateX(350); box.setTranslateY(150); box.setTranslateZ(50); //Setting the text Text text = new Text("Type any letter to rotate the box, and click on the box to stop the rotation"); //Setting the font of the text text.setFont(Font.font(null, FontWeight.BOLD, 15)); //Setting the color of the text text.setFill(Color.CRIMSON); //setting the position of the text text.setX(20); text.setY(50); //Setting the material of the box PhongMaterial material = new PhongMaterial(); material.setDiffuseColor(Color.DARKSLATEBLUE); //Setting the diffuse color material to box box.setMaterial(material); //Setting the rotation animation to the box RotateTransition rotateTransition = new RotateTransition(); //Setting the duration for the transition rotateTransition.setDuration(Duration.millis(1000)); //Setting the node for the transition rotateTransition.setNode(box); //Setting the axis of the rotation rotateTransition.setAxis(Rotate.Y_AXIS); //Setting the angle of the rotation rotateTransition.setByAngle(360); //Setting the cycle count for the transition rotateTransition.setCycleCount(50); //Setting auto reverse value to false rotateTransition.setAutoReverse(false); //Creating a text filed TextField textField = new TextField(); //Setting the position of the text field textField.setLayoutX(50); textField.setLayoutY(100); //Handling the key typed event EventHandler<KeyEvent> eventHandlerTextField = new EventHandler<KeyEvent>() { @Override public void handle(KeyEvent event) { //Playing the animation rotateTransition.play(); } }; //Adding an event handler to the text feld textField.addEventHandler(KeyEvent.KEY_TYPED, eventHandlerTextField); //Handling the mouse clicked event(on box) EventHandler<javafx.scene.input.MouseEvent> eventHandlerBox = new EventHandler<javafx.scene.input.MouseEvent>() { @Override public void handle(javafx.scene.input.MouseEvent e) { rotateTransition.stop(); } }; //Adding the event handler to the box box.addEventHandler(javafx.scene.input.MouseEvent.MOUSE_CLICKED, eventHandlerBox); //Creating a Group object Group root = new Group(box, textField, text); //Creating a scene object Scene scene = new Scene(root, 600, 300); //Setting camera PerspectiveCamera camera = new PerspectiveCamera(false); camera.setTranslateX(0); camera.setTranslateY(0); camera.setTranslateZ(0); scene.setCamera(camera); //Setting title to the Stage stage.setTitle("Event Handlers Example"); //Adding scene to the stage stage.setScene(scene); //Displaying the contents of the stage stage.show(); } public static void main(String args[]){ launch(args); } }Compile and execute the saved java file from the command prompt using the following commands.
javac EventHandlersExample.java java EventHandlersExampleOn executing, the above program generates a JavaFX window displaying a text field and a 3D box as shown below −
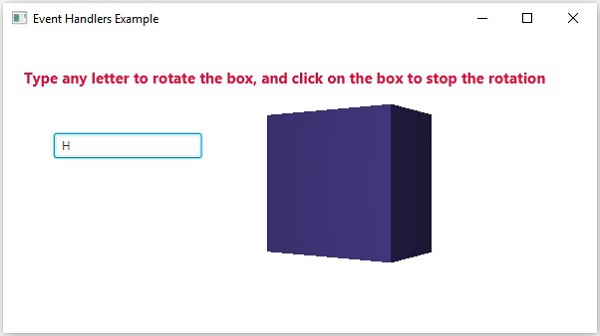
Using Convenience Methods for Event Handling
Some of the classes in JavaFX define event handler properties. By setting the values to these properties using their respective setter methods, you can register to an event handler. These methods are known as convenience methods.Most of these methods exist in the classes like Node, Scene, Window, etc., and they are available to all their sub classes.
For example, to add a mouse event listener to a button, you can use the convenience method setOnMouseClicked() as shown below.
playButton.setOnMouseClicked((new EventHandler<MouseEvent>() { public void handle(MouseEvent event) { System.out.println("Hello World"); pathTransition.play(); } }));
Example
The following program is an example that demonstrates the event handling in JavaFX using the convenience methods.Save this code in a file with the name ConvinienceMethodsExample.java.
import javafx.animation.PathTransition; import javafx.application.Application; import static javafx.application.Application.launch; import javafx.event.EventHandler; import javafx.scene.Group; import javafx.scene.Scene; import javafx.scene.control.Button; import javafx.scene.input.MouseEvent; import javafx.scene.paint.Color; import javafx.scene.shape.Circle; import javafx.scene.shape.LineTo; import javafx.scene.shape.MoveTo; import javafx.scene.shape.Path; import javafx.stage.Stage; import javafx.util.Duration; public class ConvinienceMethodsExample extends Application { @Override public void start(Stage stage) { //Drawing a Circle Circle circle = new Circle(); //Setting the position of the circle circle.setCenterX(300.0f); circle.setCenterY(135.0f); //Setting the radius of the circle circle.setRadius(25.0f); //Setting the color of the circle circle.setFill(Color.BROWN); //Setting the stroke width of the circle circle.setStrokeWidth(20); //Creating a Path Path path = new Path(); //Moving to the staring point MoveTo moveTo = new MoveTo(208, 71); //Creating 1st line LineTo line1 = new LineTo(421, 161); //Creating 2nd line LineTo line2 = new LineTo(226,232); //Creating 3rd line LineTo line3 = new LineTo(332,52); //Creating 4th line LineTo line4 = new LineTo(369, 250); //Creating 5th line LineTo line5 = new LineTo(208, 71); //Adding all the elements to the path path.getElements().add(moveTo); path.getElements().addAll(line1, line2, line3, line4, line5); //Creating the path transition PathTransition pathTransition = new PathTransition(); //Setting the duration of the transition pathTransition.setDuration(Duration.millis(1000)); //Setting the node for the transition pathTransition.setNode(circle); //Setting the path for the transition pathTransition.setPath(path); //Setting the orientation of the path pathTransition.setOrientation( PathTransition.OrientationType.ORTHOGONAL_TO_TAN GENT); //Setting the cycle count for the transition pathTransition.setCycleCount(50); //Setting auto reverse value to true pathTransition.setAutoReverse(false); //Creating play button Button playButton = new Button("Play"); playButton.setLayoutX(300); playButton.setLayoutY(250); circle.setOnMouseClicked (new EventHandler<javafx.scene.input.MouseEvent>() { @Override public void handle(javafx.scene.input.MouseEvent e) { System.out.println("Hello World"); circle.setFill(Color.DARKSLATEBLUE); } }); playButton.setOnMouseClicked((new EventHandler<MouseEvent>() { public void handle(MouseEvent event) { System.out.println("Hello World"); pathTransition.play(); } })); //Creating stop button Button stopButton = new Button("stop"); stopButton.setLayoutX(250); stopButton.setLayoutY(250); stopButton.setOnMouseClicked((new EventHandler<MouseEvent>() { public void handle(MouseEvent event) { System.out.println("Hello World"); pathTransition.stop(); } })); //Creating a Group object Group root = new Group(circle, playButton, stopButton); //Creating a scene object Scene scene = new Scene(root, 600, 300); scene.setFill(Color.LAVENDER); //Setting title to the Stage stage.setTitle("Convenience Methods Example"); //Adding scene to the stage stage.setScene(scene); //Displaying the contents of the stage stage.show(); } public static void main(String args[]){ launch(args); } }Compile and execute the saved java file from the command prompt using the following commands.
javac ConvinienceMethodsExample.java java ConvinienceMethodsExampleOn executing, the above program generates a JavaFX window as shown below. Here click on the play button to start the animation and click on the stop button to stop the animation.
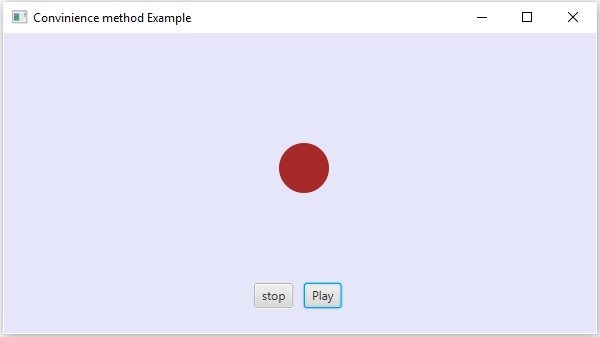
No comments:
Post a Comment