I18N (Internationalization) is the process of designing an application that can be adapted to various languages. Yii offers a full spectrum of I18N features.
Locale is a set of parameters that specify a user's language and country. For example, the en-US stands for the English locale and the United States.
Yii provides two types of languages: source language and target language. The source language is the language in which all text messages in the application are written. The target language is the language that should be used to display content to end users.
The message translation component translates text messages from the source language to the target language. To translate the message, the message translation service must look it up in a message source.
To use the message translation service, you should −
Step 2 − Now, modify the config/web.php file.
Step 3 − Now, create the messages/ru-RU directory structure. Inside the ru-RU folder create a file called app.php. This will store all EN → RU translations.
The message was translated into Russian as we set the target language to ru-RU. We can dynamically change the language of the application.
Step 6 − Modify the actionTranslation() method.
Step 7 − In a translated message, you can insert one or multiple parameters.
You can translate a whole view script, instead of translating individual text messages. For example, if the target language is ru-RU and you want to translate the views/site/index.php view file, you should translate the view and save it under the views/site/ru-RU directory.
Step 8 − Create the views/site/ru-RU directory structure. Then, inside the ru-RU folder create a file called index.php with the following code.

Locale is a set of parameters that specify a user's language and country. For example, the en-US stands for the English locale and the United States.
Yii provides two types of languages: source language and target language. The source language is the language in which all text messages in the application are written. The target language is the language that should be used to display content to end users.
The message translation component translates text messages from the source language to the target language. To translate the message, the message translation service must look it up in a message source.
To use the message translation service, you should −
- Wrap text messages you want to be translated in the Yii::t() method.
- Configure message sources.
- Store messages in the message source.
echo \Yii::t('app', 'This is a message to translate!');In the above code snippet, the 'app' stands for a message category.
Step 2 − Now, modify the config/web.php file.
<?php $params = require(__DIR__ . '/params.php'); $config = [ 'id' => 'basic', 'basePath' => dirname(__DIR__), 'bootstrap' => ['log'], 'components' => [ 'request' => [ // !!! insert a secret key in the following (if it is empty) - this //is required by cookie validation 'cookieValidationKey' => 'ymoaYrebZHa8gURuolioHGlK8fLXCKjO', ], 'cache' => [ 'class' => 'yii\caching\FileCache', ], 'i18n' => [ 'translations' => [ 'app*' => [ 'class' => 'yii\i18n\PhpMessageSource', 'fileMap' => [ 'app' => 'app.php' ], ], ], ], 'user' => [ 'identityClass' => 'app\models\User', 'enableAutoLogin' => true, ], 'errorHandler' => [ 'errorAction' => 'site/error', ], 'mailer' => [ 'class' => 'yii\swiftmailer\Mailer', // send all mails to a file by default. You have to set // 'useFileTransport' to false and configure a transport // for the mailer to send real emails. 'useFileTransport' => true, ], 'log' => [ 'flushInterval' => 1, 'traceLevel' => YII_DEBUG ? 3 : 0, 'targets' => [ [ 'class' => 'yii\log\FileTarget', 'exportInterval' => 1, 'logVars' => [], ], ], ], 'db' => require(__DIR__ . '/db.php'), ], // set target language to be Russian 'language' => 'ru-RU', // set source language to be English 'sourceLanguage' => 'en-US', 'modules' => [ 'hello' => [ 'class' => 'app\modules\hello\Hello', ], ], 'params' => $params, ]; if (YII_ENV_DEV) { // configuration adjustments for 'dev' environment $config['bootstrap'][] = 'debug'; $config['modules']['debug'] = [ 'class' => 'yii\debug\Module', ]; $config['bootstrap'][] = 'gii'; $config['modules']['gii'] = [ 'class' => 'yii\gii\Module', ]; } return $config; ?>In the above code, we define the source and the target languages. We also specify a message source supported by yii\i18n\PhpMessageSource. The app* pattern indicates that all messages categories starting with app must be translated using this particular message source. In the above configuration, all Russian translations will be located in the messages/ru-RU/app.php file.
Step 3 − Now, create the messages/ru-RU directory structure. Inside the ru-RU folder create a file called app.php. This will store all EN → RU translations.
<?php return [ 'This is a string to translate!' => 'Эта строка для перевода!' ]; ?>Step 4 − Create a function called actionTranslation() in the SiteController.
public function actionTranslation() { echo \Yii::t('app', 'This is a string to translate!'); }Step 5 − Enter the URL http://localhost:8080/index.php?r=site/translation in the web browser, you will see the following.
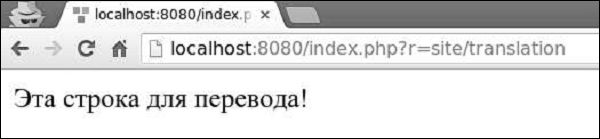
Step 6 − Modify the actionTranslation() method.
public function actionTranslation() { \Yii::$app->language = 'en-US'; echo \Yii::t('app', 'This is a string to translate!'); }Now, the message is displayed in English −
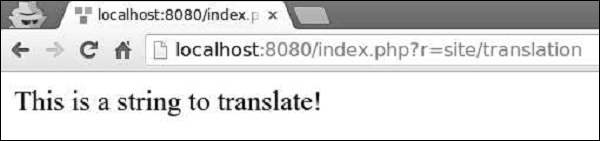
public function actionTranslation() { $username = 'Vladimir'; // display a translated message with username being "Vladimir" echo \Yii::t('app', 'Hello, {username}!', [ 'username' => $username, ]), "<br>"; $username = 'John'; // display a translated message with username being "John" echo \Yii::t('app', 'Hello, {username}!', [ 'username' => $username, ]), "<br>"; $price = 150; $count = 3; $subtotal = 450; echo \Yii::t('app', 'Price: {0}, Count: {1}, Subtotal: {2}', [$price, $count, $subtotal]); }Following will be the output.

Step 8 − Create the views/site/ru-RU directory structure. Then, inside the ru-RU folder create a file called index.php with the following code.
<?php /* @var $this yii\web\View */ $this->title = 'My Yii Application'; ?> <div class = "site-index"> <div class = "jumbotron"> <h1>Добро пожаловать!</h1> </div> </div>Step 9 − The target language is ru-RU, so if you enter the URL http://localhost:8080/index.php?r=site/index, you will see the page with Russian translation.

No comments:
Post a Comment