Cascading Style Sheets, also referred to as CSS, is a simple design language intended to simplify the process of making web pages presentable.
CSS handles the look and feel part of a web page. Using CSS, you can control the color of the text, style of fonts, spacing between paragraphs, size of columns and layout.
Apart from these, you can also control the background images or colors that are used, layout designs, variations in display for different devices and screen sizes as well as a variety of other effects.
A CSS comprises of style rules that are interpreted by the browser and then applied to the corresponding elements in your document.
A style rule is made of three parts, which are −
The default style sheet used by JavaFX is modena.css. It is found in the JavaFX runtime jar.
The following program is an example which demonstrates how to add styles to the above application in JavaFX.
Save this code in a file with the name CssExample.java
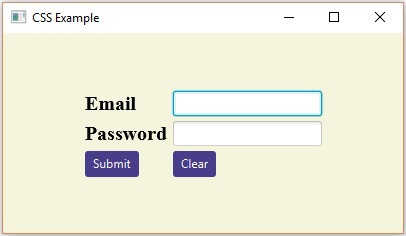
CSS handles the look and feel part of a web page. Using CSS, you can control the color of the text, style of fonts, spacing between paragraphs, size of columns and layout.
Apart from these, you can also control the background images or colors that are used, layout designs, variations in display for different devices and screen sizes as well as a variety of other effects.
CSS in JavaFX
JavaFX provides you the facility of using CSS to enhance the look and feel of the application. The package javafx.css contains the classes that are used to apply CSS for JavaFX applications.A CSS comprises of style rules that are interpreted by the browser and then applied to the corresponding elements in your document.
A style rule is made of three parts, which are −
- Selector − A selector is an HTML tag at which a style will be applied. This could be any tag like <h1> or <table>, etc.
- Property − A property is a type of attribute of the HTML tag. In simpler terms, all the HTML attributes are converted into CSS properties. They could be color, border, etc.
- Value − Values are assigned to properties. For example, a color property can have value either red or #F1F1F1, etc.
selector { property: value }
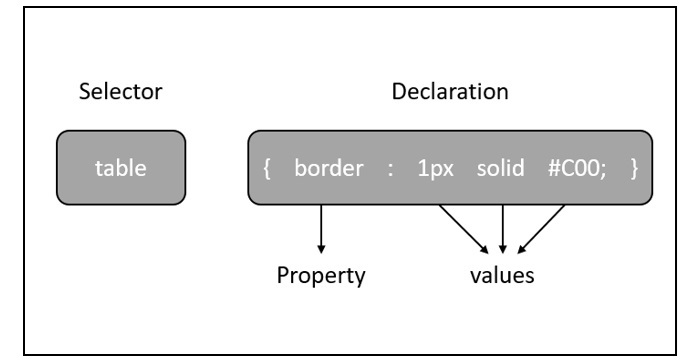
Adding Your own Style Sheet
You can add your own style sheet to a scene in JavaFX as follows −Scene scene = new Scene(new Group(), 500, 400); scene.getStylesheets().add("path/stylesheet.css");
Adding Inline Style Sheets
You can also add in-line styles using the setStyle() method. These styles consist of only key-value pairs and they are applicable to the nodes on which they are set. Following is a sample code of setting an inline style sheet to a button..button { -fx-background-color: red; -fx-text-fill: white; }
Example
Assume that we have developed an JavaFX application which displays a form with a Text Field, Password Field, Two Buttons. By default, this form looks as shown in the following screenshot −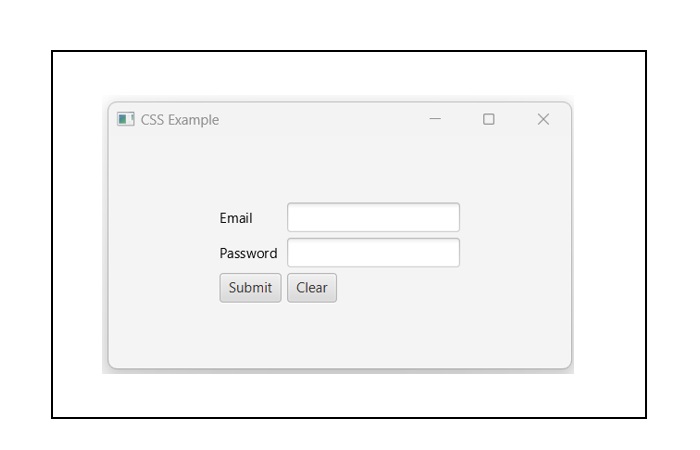
Save this code in a file with the name CssExample.java
import javafx.application.Application; import static javafx.application.Application.launch; import javafx.geometry.Insets; import javafx.geometry.Pos; import javafx.scene.Scene; import javafx.scene.control.Button; import javafx.scene.control.PasswordField; import javafx.scene.layout.GridPane; import javafx.scene.text.Text; import javafx.scene.control.TextField; import javafx.stage.Stage; public class CssExample extends Application { @Override public void start(Stage stage) { //creating label email Text text1 = new Text("Email"); //creating label password Text text2 = new Text("Password"); //Creating Text Filed for email TextField textField1 = new TextField(); //Creating Text Filed for password PasswordField textField2 = new PasswordField(); //Creating Buttons Button button1 = new Button("Submit"); Button button2 = new Button("Clear"); //Creating a Grid Pane GridPane gridPane = new GridPane(); //Setting size for the pane gridPane.setMinSize(400, 200); //Setting the padding gridPane.setPadding(new Insets(10, 10, 10, 10)); //Setting the vertical and horizontal gaps between the columns gridPane.setVgap(5); gridPane.setHgap(5); //Setting the Grid alignment gridPane.setAlignment(Pos.CENTER); //Arranging all the nodes in the grid gridPane.add(text1, 0, 0); gridPane.add(textField1, 1, 0); gridPane.add(text2, 0, 1); gridPane.add(textField2, 1, 1); gridPane.add(button1, 0, 2); gridPane.add(button2, 1, 2); //Styling nodes button1.setStyle("-fx-background-color: darkslateblue; -fx-text-fill: white;"); button2.setStyle("-fx-background-color: darkslateblue; -fx-text-fill: white;"); text1.setStyle("-fx-font: normal bold 20px 'serif' "); text2.setStyle("-fx-font: normal bold 20px 'serif' "); gridPane.setStyle("-fx-background-color: BEIGE;"); // Creating a scene object Scene scene = new Scene(gridPane); // Setting title to the Stage stage.setTitle("CSS Example"); // Adding scene to the stage stage.setScene(scene); //Displaying the contents of the stage stage.show(); } public static void main(String args[]){ launch(args); } }Compile and execute the saved java file from the command prompt using the following commands.
javac CssExample.java java CssExampleOn executing, the above program generates a JavaFX window as shown below.
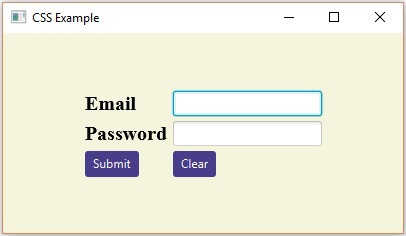
No comments:
Post a Comment